This C program demonstrates how to print a semicolon without using the semicolon character itself.
Problem Statement
Write a C program that takes a sentence as input and reverses the order of the words in the sentence. The program should output the modified sentence with the reversed word order.
The program should adhere to the following specifications:
- The maximum length of the input sentence should be limited to 100 characters.
- The words in the sentence are separated by spaces.
- The program should handle leading and trailing spaces in the input sentence.
- The program should not modify the individual words within the sentence, only their order.
C Program to Print Semicolon without using Semicolon
#include <stdio.h> #define MAX_LENGTH 100 void reverseWords(char* sentence) { char reversedSentence[MAX_LENGTH]; int i, j, wordEnd, reversedIndex; // Initialize variables wordEnd = reversedIndex = MAX_LENGTH - 1; // Iterate through the characters in the sentence for (i = MAX_LENGTH - 1; i >= 0; i--) { if (sentence[i] != ' ' && (i == 0 || sentence[i - 1] == ' ')) { // Copy the word from the input sentence to the reversed sentence for (j = i; j <= wordEnd; j++) { reversedSentence[reversedIndex--] = sentence[j]; } // Update the wordEnd to the position before the space wordEnd = i - 1; } } // Terminate the reversed sentence with a null character reversedSentence[MAX_LENGTH - 1 - reversedIndex] = '\0'; // Print the reversed sentence printf("Reversed sentence: %s\n", reversedSentence); } int main() { char sentence[MAX_LENGTH]; printf("Enter a sentence (max %d characters): ", MAX_LENGTH - 1); fgets(sentence, sizeof(sentence), stdin); reverseWords(sentence); return 0; }
How it Works
- Read the input sentence from the user, limiting it to a maximum of 100 characters.
- Create an array to store the reversed sentence, with the same size as the input sentence.
- Initialize variables to keep track of the current word’s start and end positions in the input sentence and the reversed sentence.
- Starting from the end of the input sentence, iterate backward through each character.
- If the current character is not a space and the previous character is a space (or it is the first character), it means we have reached the start of a new word.
- Assign the current position as the end of the word in the input sentence.
- Copy the characters of the word from the input sentence to the reversed sentence, starting from the current position in the reversed sentence and moving backward.
- Update the current position in the reversed sentence to the next available position.
- After processing all the characters in the input sentence, terminate the reversed sentence with a null character.
- Print the reversed sentence as the output.
Input /Output
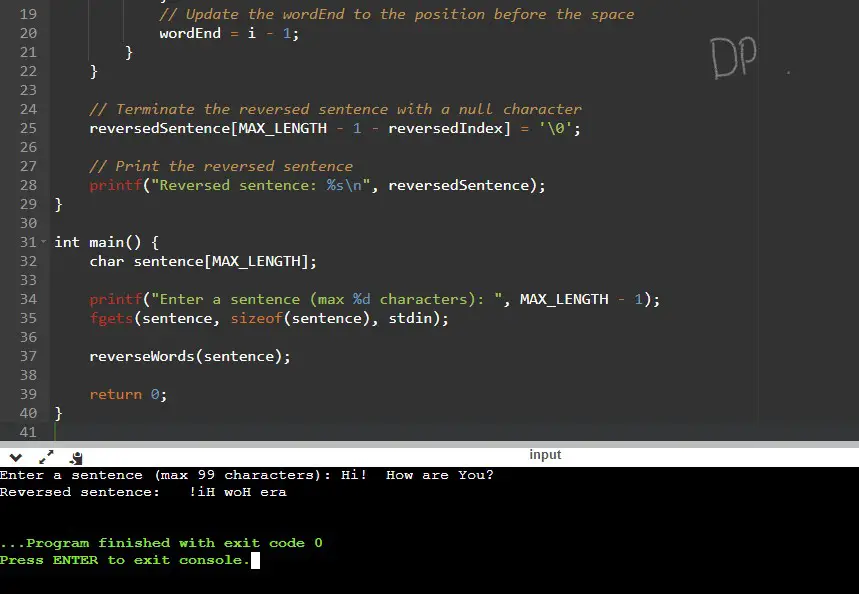