This C program converts a binary number to its corresponding Gray code using iterative methods.
Gray code, also known as reflected binary code, is a binary numeral system where adjacent values differ in only one bit position. It finds applications in various areas such as analog-to-digital converters, error detection, and rotary encoders. Unlike traditional binary representation, Gray code ensures that only one bit changes at a time during transitions between consecutive values.
Problem Statement
The program should allow the user to input a binary number and output its Gray code, as well as input a Gray code and output its corresponding binary number. The program should not use recursion for the conversion process.
Example:
Input: Enter a binary number: 1010
Output: Gray code: 523 Binary code: 1010
Note: The program should handle valid input values within the range of unsigned integers.
C Program to Convert Binary to Gray Code without Recursion
#include <stdio.h> // Function to convert binary to gray code unsigned int binaryToGray(unsigned int num) { return (num >> 1) ^ num; } // Function to convert gray code to binary unsigned int grayToBinary(unsigned int num) { unsigned int mask; for (mask = num >> 1; mask != 0; mask = mask >> 1) { num = num ^ mask; } return num; } int main() { unsigned int binary, gray; printf("Enter a binary number: "); scanf("%u", &binary); gray = binaryToGray(binary); printf("Gray code: %u\n", gray); binary = grayToBinary(gray); printf("Binary code: %u\n", binary); return 0; }
How it Works
The C program works by implementing two functions: binaryToGray()
and grayToBinary()
, which handle the conversion between binary and Gray code.
binaryToGray()
Function:- It takes an unsigned integer
num
as input, which represents a binary number. - Inside the function, the binary-to-Gray conversion is performed using the following steps:
- The
num
value is right-shifted by 1 (num >> 1
), which moves all the bits one position to the right. - The result of the right shift is then XORed with the original
num
value (num ^ (num >> 1)
). - The XOR operation between the shifted and original number generates the Gray code representation of the binary number.
- The
- The Gray code value is returned as the output of the function.
- It takes an unsigned integer
grayToBinary()
Function:- It takes an unsigned integer
num
as input, which represents a Gray code. - Inside the function, the Gray-to-binary conversion is performed using the following steps:
- A mask variable is initialized as
num >> 1
, which shifts thenum
value one position to the right. - A loop is executed until the mask becomes zero:
- Inside the loop, the
num
value is XORed with the mask (num ^ mask
). - The result is stored back in the
num
variable. - The mask is right-shifted by 1 (
mask = mask >> 1
).
- Inside the loop, the
- After the loop completes, the final
num
value represents the binary representation of the Gray code.
- A mask variable is initialized as
- The binary code value is returned as the output of the function.
- It takes an unsigned integer
main()
Function:- The
main()
function is the entry point of the program. - It prompts the user to enter a binary number.
- The binary number is read from the user using the
scanf()
function and stored in the variablebinary
. - The
binaryToGray()
function is called with thebinary
value to convert it to Gray code, and the result is stored in the variablegray
. - The Gray code value is displayed as output using
printf()
. - The
grayToBinary()
function is called with thegray
value to convert it back to binary, and the result is stored in the variablebinary
. - The binary code value is displayed as output using
printf()
.
- The
The program follows the same process for each conversion scenario, allowing the user to input a binary number and obtain its corresponding Gray code, or input a Gray code and obtain its corresponding binary number.
Input /Output
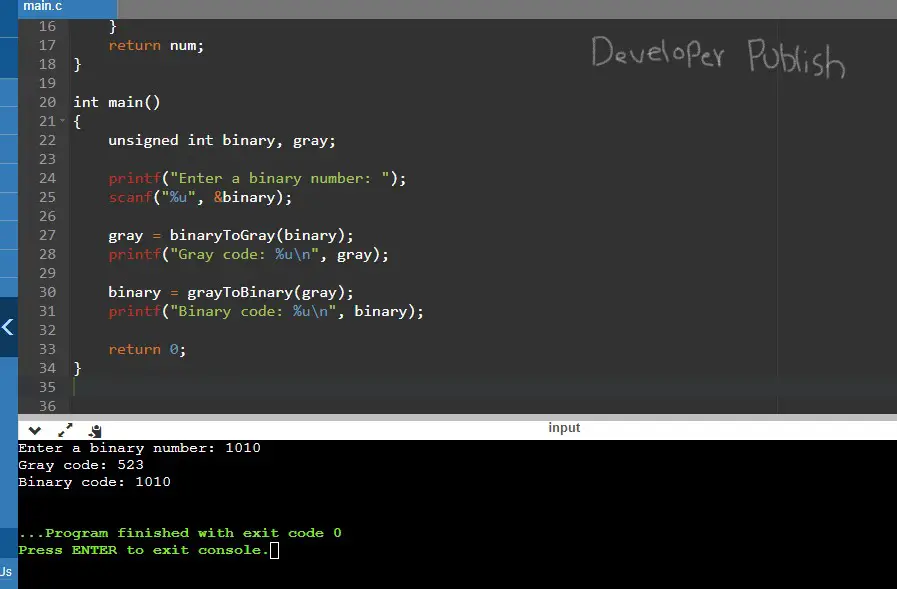