This C program finds the first and last occurrence of a target character in a given string and displays their indices.
Problem Statement
Given a string and a target character, we need to find and display the indices of the first and last occurrences of the target character in the string. If the target character is not found in the string, an appropriate message should be displayed.
C Program to Find First and Last Occurrence of Character in a String
#include <stdio.h> #include <string.h> void findFirstAndLastOccurrence(char str[], char ch) { int len = strlen(str); int first = -1; int last = -1; for (int i = 0; i < len; i++) { if (str[i] == ch) { if (first == -1) { first = i; } last = i; } } if (first != -1 && last != -1) { printf("First occurrence: %d\n", first); printf("Last occurrence: %d\n", last); } else { printf("Character not found in the string.\n"); } } int main() { char str[100]; char ch; printf("Enter a string: "); fgets(str, sizeof(str), stdin); printf("Enter a character to find: "); scanf("%c", &ch); findFirstAndLastOccurrence(str, ch); return 0; }
How it Works
- The program starts by including the necessary header files:
stdio.h
for input/output operations andstring.h
for string manipulation functions. - The program defines a function named
findFirstAndLastOccurrence
that takes two parameters: the input stringstr
and the character to findch
. - Inside the
findFirstAndLastOccurrence
function, the length of the stringstr
is calculated using thestrlen
function from thestring.h
library. This length is stored in the variablelen
. - Two variables,
first
andlast
, are initialized to -1. These variables will keep track of the indices of the first and last occurrences of the characterch
in the string. - A loop is used to iterate through each character of the string. The loop starts from index 0 and continues until it reaches the end of the string.
- Inside the loop, each character of the string is checked against the target character
ch
. If a match is found, the program updates thefirst
variable if it hasn’t been set yet and updates thelast
variable regardless. - After the loop, the program checks whether both
first
andlast
variables have been updated. If they have been updated, it means that the characterch
was found in the string. In this case, the program prints the indices of the first and last occurrences using theprintf
function. - If the
first
orlast
variables haven’t been updated, it means that the characterch
was not found in the string. In this case, the program prints a message indicating that the character was not found using theprintf
function. - In the
main
function, the program starts by declaring the necessary variables:str
to hold the input string andch
to store the target character. - The user is prompted to enter a string using the
printf
function. Thefgets
function is then used to read the input string from the user. - Next, the user is prompted to enter the character to find using another
printf
function. Thescanf
function is used to read the character. - Finally, the
findFirstAndLastOccurrence
function is called with the provided string and character as arguments. - The program ends by returning 0 from the
main
function.
By following these steps, the program takes input from the user, searches for the first and last occurrences of a given character in the input string, and displays the indices of the first and last occurrences accordingly.
Input / Output
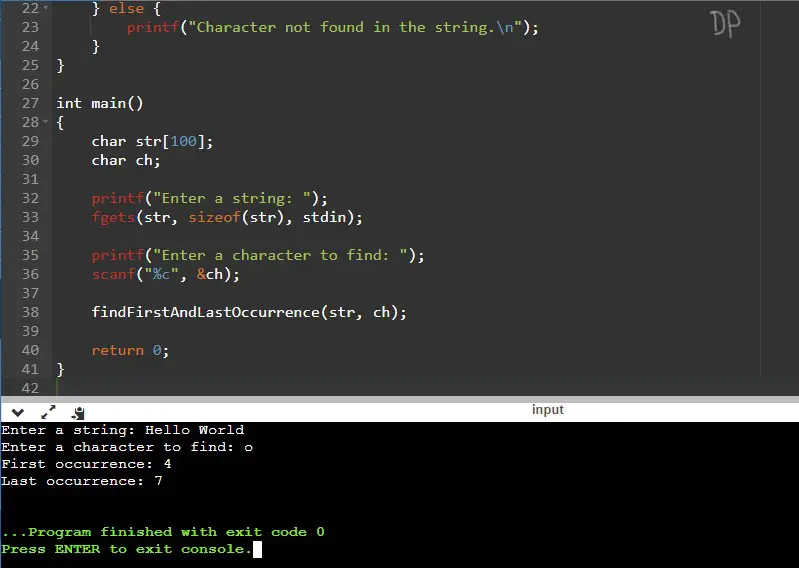