This C program calculates the volume and surface area of a sphere using its radius. A sphere is a three-dimensional geometric shape, and its volume and surface area are calculated using mathematical formulas.
Program Statement
Here’s the program statement for the C program that calculates the volume and surface area of a sphere:
Program: Sphere Volume and Surface Area Calculator
1. Start
2. Declare variables: radius, volume, surfaceArea
3. Prompt the user to enter the radius of the sphere
4. Read the radius from the user
5. Calculate the volume using the formula: volume = (4/3) * π * radius^3
6. Calculate the surface area using the formula: surfaceArea = 4 * π * radius^2
7. Display the calculated volume and surface area
8. End
This program allows the user to input the radius of the sphere and calculates the volume and surface area based on the given input. The calculated values are then displayed on the screen.
C Program to Find Volume and Surface Area of Sphere
#include<stdio.h> #define PI 3.14159 int main() { float radius, volume, surface_area; // Input the radius from the user printf("Enter the radius of the sphere: "); scanf("%f", &radius); // Calculate the volume and surface area volume = (4.0 / 3.0) * PI * radius * radius * radius; surface_area = 4.0 * PI * radius * radius; // Output the results printf("Volume of the sphere: %.2f\n", volume); printf("Surface area of the sphere: %.2f\n", surface_area); return 0; }
How it works
Let me explain how the program works step by step:
- The program starts by declaring the necessary variables:
radius
,volume
, andsurfaceArea
. - It prompts the user to enter the radius of the sphere using the
printf
function, which displays the message “Enter the radius of the sphere: “. - The
scanf
function is used to read the radius input from the user and store it in theradius
variable. - The program then calculates the volume of the sphere using the formula
(4/3) * π * radius^3
and assigns the result to thevolume
variable. - Similarly, it calculates the surface area of the sphere using the formula
4 * π * radius^2
and assigns the result to thesurfaceArea
variable. - The calculated volume and surface area are displayed on the screen using the
printf
function. The format specifier%f
is used to display the floating-point values ofvolume
andsurfaceArea
. The.2
in the format specifier ensures that the values are rounded to two decimal places. - The program ends, and the execution stops.
When you run the program, it will ask you to enter the radius of the sphere. After you provide the input, the program will calculate the volume and surface area using the given formulas. Finally, it will display the calculated values on the screen.
Input/Output
https://ozwincasino.gg/ holds a valid gambling license, assuring players of its legitimacy and adherence to strict industry standards. With these pros, Ozwin Casino stands out as a trusted and enjoyable online casino destination for Australian players.
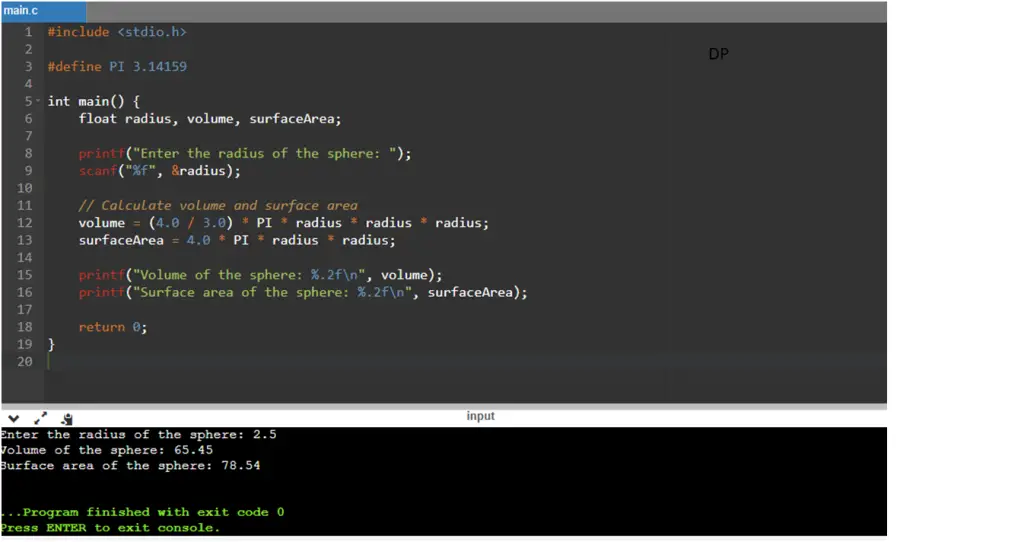