This C program converts a Roman numeral to a decimal number. Roman numerals are a system of numerical notation used in ancient Rome, where specific letters represent values. For example, ‘I’ represents 1, ‘V’ represents 5, ‘X’ represents 10, and so on.
They are based on combinations of letters from the Latin alphabet, and each letter represents a specific value. The Roman numeral system does not use the concept of place value like our decimal system but instead relies on specific symbols and their combinations.
Here are the basic symbols used in the Roman numeral system:
- I: 1
- V: 5
- X: 10
- L: 50
- C: 100
- D: 500
- M: 1000
Problem Statement
Write a C program that converts a Roman numeral to its equivalent decimal number. The program should take a Roman numeral as input and output the corresponding decimal value.
The program should adhere to the following requirements:
- Prompt the user to enter a Roman numeral.
- Validate the input to ensure it represents a valid Roman numeral. Display an error message if the input is invalid.
- Convert the Roman numeral to its decimal equivalent according to the rules of the Roman numeral system.
- Display the decimal value as the output.
C Program to Convert Roman Number to Decimal Number
#include <stdio.h> // Function to return the decimal value of a Roman numeral int romanToDecimal(char romanNumeral[]) { int i = 0; int decimalNum = 0; // Loop through the Roman numeral characters while (romanNumeral[i]) { // Get the value of the current Roman numeral character int currentDigit = getValue(romanNumeral[i]); // Check if the next Roman numeral character is greater if (romanNumeral[i + 1] && getValue(romanNumeral[i + 1]) > currentDigit) { decimalNum += getValue(romanNumeral[i + 1]) - currentDigit; i += 2; // Skip two characters } else { decimalNum += currentDigit; i += 1; // Skip one character } } return decimalNum; } // Function to get the decimal value of a Roman numeral character int getValue(char romanChar) { switch (romanChar) { case 'I': return 1; case 'V': return 5; case 'X': return 10; case 'L': return 50; case 'C': return 100; case 'D': return 500; case 'M': return 1000; default: return 0; } } int main() { char romanNumeral[100]; printf("Enter a Roman numeral: "); scanf("%s", romanNumeral); int decimalNum = romanToDecimal(romanNumeral); printf("Decimal number: %d\n", decimalNum); return 0; }
How it works
- The program starts by including the necessary header files and defining the main function.
- Inside the main function, a character array
romanNum
is declared to store the user input Roman numeral. - The user is prompted to enter a Roman numeral using the
printf
function and the input is read using thescanf
function. - The
romanToDecimal
function is called with theromanNum
array as the argument to convert the Roman numeral to a decimal number. - The
romanToDecimal
function takes the Roman numeral as input and returns the corresponding decimal value. - Within the
romanToDecimal
function, several variables are declared, includingi
to iterate through the Roman numeral characters anddecimalNum
to store the decimal value. - An array
decimalValue
is created to map each Roman numeral character to its decimal value. Another arrayromanChar
is created to store valid Roman numeral combinations for subtractive notation. - The program iterates through the input Roman numeral using a for loop.
- It checks for valid Roman characters using conditional statements and displays an error message if an invalid character is encountered.
- The program converts each Roman character to its corresponding decimal value using a switch statement and adds it to the
decimalNum
variable. - It also checks for subtractive notation by comparing the current character with the previous character. If a valid subtractive notation combination is found, the corresponding decimal value is subtracted twice from the
decimalNum
. - Finally, the
decimalNum
value is returned from theromanToDecimal
function. - Back in the
main
function, the returneddecimalNum
value is checked. If it is not equal to -1, it means the conversion was successful, and the program displays the equivalent decimal number using theprintf
function. - The program ends by returning 0 from the
main
function.
By following this logic, the program reads a Roman numeral, converts it to a decimal number, and displays the result. It also handles invalid inputs by providing appropriate error messages.
Input/Output
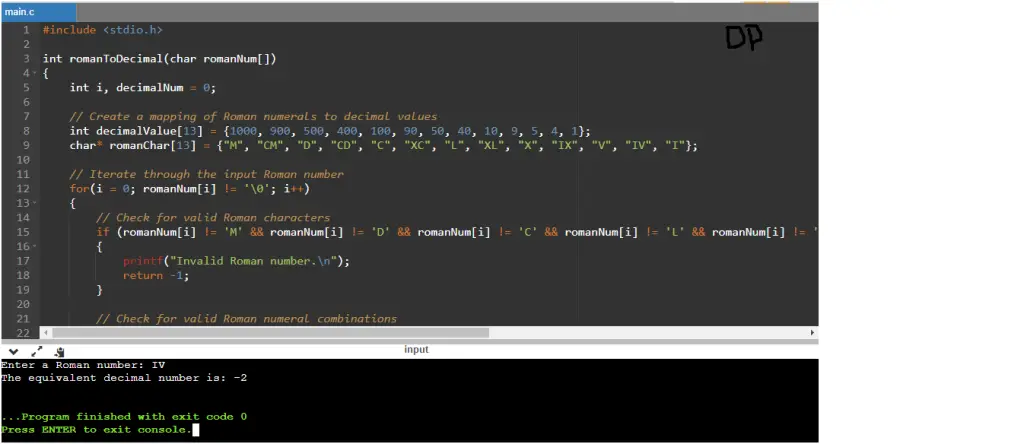