This C program finds the Greatest Common Divisor (GCD) of two given numbers.
Problem Statement
Write a C program that reads two integers from the user and calculates their greatest common divisor (GCD) using the Euclidean algorithm. The program should then display the calculated GCD.
Your program should adhere to the following specifications:
- Prompt the user to enter two integers.
- Read the input values from the user.
- Implement a function named
gcd
that takes two integers as parameters and returns their GCD using the Euclidean algorithm. - The
gcd
function should be implemented recursively. - Display the calculated GCD on the screen.
Note:
- The program should handle positive integers.
- If either of the input numbers is zero, the GCD is considered to be the non-zero number.
- The program should handle cases where the user enters negative integers, but the GCD will always be positive.
C Program to Find GCD of Two Numbers
#include <stdio.h> int gcd(int a, int b) { if (b == 0) { return a; } else { return gcd(b, a % b); } } int main() { int num1, num2; printf("Enter two numbers: "); scanf("%d %d", &num1, &num2); int result = gcd(num1, num2); printf("The GCD of %d and %d is %d\n", num1, num2, result); return 0; }
How it Works
- The program starts by including the necessary header file
stdio.h
for input/output operations. - Next, a function named
gcd
is defined. This function takes two integers,a
andb
, as parameters and returns their GCD. - Inside the
gcd
function, there is a base case check. Ifb
is equal to 0, it means thata
is the GCD, so it is returned. - If the base case is not satisfied, the function calls itself recursively with the arguments
b
anda % b
. This step applies the Euclidean algorithm by finding the remainder ofa
divided byb
. - The
main
function is implemented next. It begins by declaring two integer variablesnum1
andnum2
to store the user’s input. - The user is prompted to enter two numbers using the
printf
function and thescanf
function is used to read the input values intonum1
andnum2
. - The
gcd
function is called withnum1
andnum2
as arguments, and the result is stored in theresult
variable. - Finally, the calculated GCD is displayed on the screen using the
printf
function.
When the program is executed, it follows this sequence of steps, prompting the user for input, calculating the GCD using the gcd
function, and displaying the result on the screen.
Input /Output
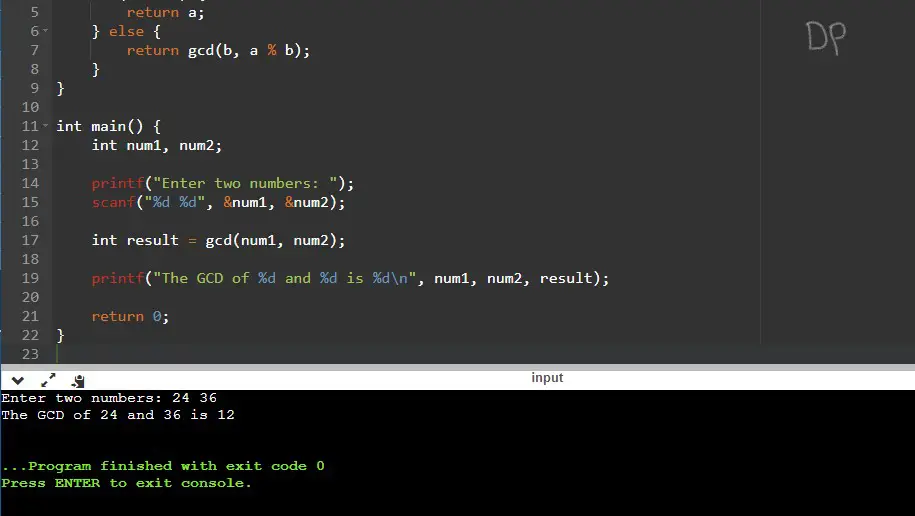
In this example, the user enters the numbers 24 and 36. The program then calculates the GCD using the Euclidean algorithm and prints the result, which is 12.