This C program finds the Least Common Multiple (LCM) of two given numbers.
Problem Statement
Write a C program that takes two positive integers as input and calculates their least common multiple (LCM). The program should output the calculated LCM.
The LCM of two numbers is the smallest positive integer that is divisible by both numbers without leaving any remainder.
Your program should have the following components:
- A function called
findGCD
that calculates the greatest common divisor (GCD) of two numbers using the Euclidean algorithm. This function should take two integers as input and return the GCD as an integer. - A function called
findLCM
that takes two integers as input and calculates the LCM using the formula: LCM = (num1 * num2) / GCD. This function should call thefindGCD
function to find the GCD and return the calculated LCM as an integer. - In the
main
function, prompt the user to enter two positive integers. - Call the
findLCM
function with the user-provided input values and store the calculated LCM in a variable. - Display the calculated LCM on the screen.
Ensure that your program handles invalid input gracefully and provides appropriate error messages if the user enters non-positive integers or non-integer values.
Note:
- You can assume that the input numbers will be positive integers.
- You can use the standard input/output functions from the
<stdio.h>
library.
C Program to Find LCM of Two Numbers
#include <stdio.h> // Function to find the GCD (Greatest Common Divisor) of two numbers int findGCD(int a, int b) { if (b == 0) { return a; } else { return findGCD(b, a % b); } } // Function to find the LCM (Least Common Multiple) of two numbers int findLCM(int a, int b) { int gcd = findGCD(a, b); int lcm = (a * b) / gcd; return lcm; } int main() { int num1, num2; printf("Enter the first number: "); scanf("%d", &num1); printf("Enter the second number: "); scanf("%d", &num2); int lcm = findLCM(num1, num2); printf("The LCM of %d and %d is %d\n", num1, num2, lcm); return 0; }
How it Works
- The program starts by defining two functions:
findGCD
andfindLCM
. These functions will be used to calculate the greatest common divisor (GCD) and the least common multiple (LCM) of two numbers, respectively. - The
findGCD
function takes two integers as input and calculates the GCD using the Euclidean algorithm. It does this by recursively calling itself with the second number and the remainder of the first number divided by the second number, until the second number becomes zero. At that point, it returns the first number, which is the GCD. - The
findLCM
function takes two integers as input. It calls thefindGCD
function to find the GCD of the two numbers. Then, it calculates the LCM using the formula: LCM = (num1 * num2) / GCD. Finally, it returns the calculated LCM as an integer. - In the
main
function, the program prompts the user to enter two positive integers. - The program reads the user input and stores the entered numbers in variables
num1
andnum2
. - It then calls the
findLCM
function withnum1
andnum2
as arguments. - The
findLCM
function calculates the LCM by finding the GCD and applying the formula. - The calculated LCM is stored in a variable called
lcm
. - Finally, the program displays the calculated LCM on the screen using the
printf
function. - The program terminates and returns 0 to indicate successful execution.
By following this step-by-step process, the program is able to calculate the LCM of the two input numbers and display the result to the user.
Input /Output
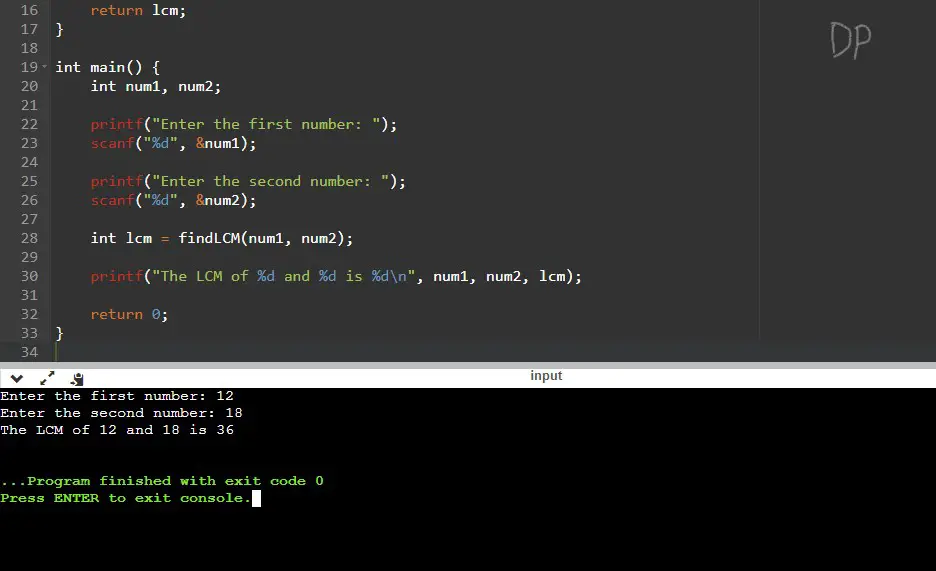
In this example, the user inputs two numbers: 12 and 18. The program then calculates the LCM of these two numbers, which is 36. Finally, the program outputs the result: “The LCM of 12 and 18 is 36.”