This C program performs cyclic permutation (rotation) of the elements in an array to the right. Cyclic permutation is an operation that moves the elements of the array in a circular fashion, where the last element becomes the first element after the rotation.
In computer programming, cyclically permuting the elements of an array involves shifting each element one position to the right, with the last element moving to the first position. This rearrangement creates a new permutation of the array while maintaining the order of the elements.
Program Statement
Write a Program in C to cyclically permute the elements of an array.
C Program to Cyclically Permute the Elements of an Array
#include <stdio.h> void cyclicallyPermute(int arr[], int size) { int temp = arr[size-1]; // Store the last element // Shift elements to the right for (int i = size-1; i > 0; i--) { arr[i] = arr[i-1]; } arr[0] = temp; // Move the last element to the first position } int main() { int arr[] = {1, 2, 3, 4, 5}; int size = sizeof(arr) / sizeof(arr[0]); printf("Original array: "); for (int i = 0; i < size; i++) { printf("%d ", arr[i]); } cyclicallyPermute(arr, size); printf("\nCyclically permuted array: "); for (int i = 0; i < size; i++) { printf("%d ", arr[i]); } return 0; }
How it works
Here’s how the program works:
- The program starts and includes the necessary header file,
stdio.h
, for input/output operations. - The
cyclicallyPermute
function is defined. It takes an arrayarr
and its sizen
as input. - Within the
cyclicallyPermute
function, a temporary variabletemp
is declared to store the last element of the array. - A
for
loop is used to iterate through the array in reverse order, starting from the second-to-last element (n-1
) and moving towards the first element (0
). - Inside the loop, each element is shifted one position to the right by assigning the value of the previous element to the current element (
arr[i] = arr[i - 1]
). - After the loop, the stored last element is placed in the first position of the array (
arr[0] = temp
). - The
displayArray
function is defined to print the elements of an array. It takes an arrayarr
and its sizen
as input. - Inside the
displayArray
function, afor
loop is used to iterate through the array elements. Each element is printed usingprintf
, followed by a space. - In the
main
function, an example arrayarr
is created with some initial values. - The size of the array
n
is calculated by dividing the total size of the array by the size of one element. - The original array is displayed using the
displayArray
function. - The
cyclicallyPermute
function is called, passing the array and its size as arguments, to perform the cyclic permutation. - Finally, the cyclically permuted array is displayed using the
displayArray
function. - The program ends, and the execution is complete.
When you run the program, it will output the original array and the cyclically permuted array, demonstrating the cyclic permutation of the array elements.
Input/Output
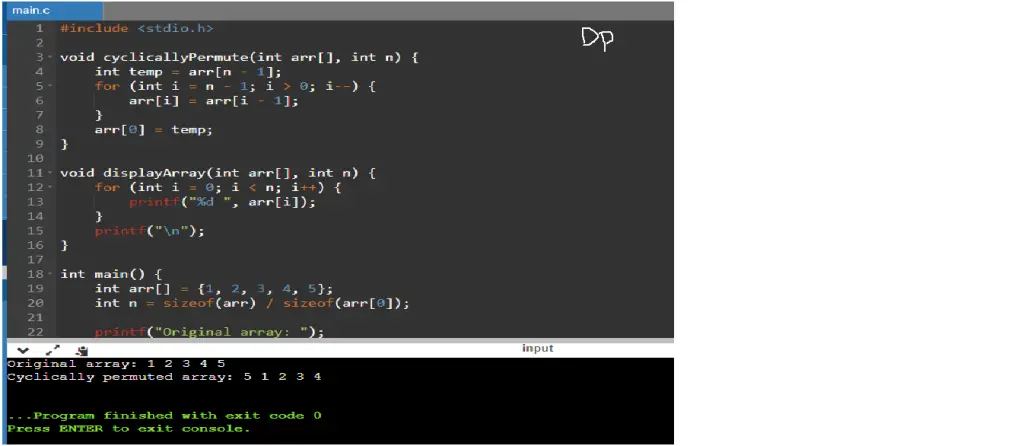