This C program checks if a specific bit position in an integer is set to one or not. In binary representation, each bit in an integer is either 0 (unset) or 1 (set). The program takes an integer and a bit position as input and determines whether the bit at that position is set to 1.
Program Statement
Write a C program that takes an integer number and a bit position as input from the user. The program should check whether the bit at the specified position in the binary representation of the number is set to one or not. If the bit is set, the program should display a message indicating that the bit is set to one. Otherwise, if the bit is unset, the program should display a message indicating that the bit is not set to one. The program should handle invalid input by checking if the bit position is within the range of 0 to 31. If the bit position is outside this range, the program should display an error message indicating an invalid bit position.
The program should follow these steps:
- Prompt the user to enter an integer number.
- Read and store the number from the user.
- Prompt the user to enter the bit position to check.
- Read and store the bit position from the user.
- Validate the bit position to ensure it is within the range of 0 to 31. If it is outside this range, display an error message and terminate the program.
- Use bitwise operations to check if the bit at the specified position in the binary representation of the number is set or unset.
- Display an appropriate message indicating whether the bit is set to one or not.
By implementing this program, you will be able to input a number and a bit position, and determine if the bit at that position is set to one or not in the binary representation of the number.
C Program to Check if Bit Position is Set to One or not
#include <stdio.h> int isBitSet(int num, int pos) { // Shift 1 to the left by the position and perform a bitwise AND operation // If the result is non-zero, the bit is set; otherwise, it is not set return (num & (1 << pos)) != 0; } int main() { int num, pos; printf("Enter an integer: "); scanf("%d", &num); printf("Enter the bit position: "); scanf("%d", &pos); if (isBitSet(num, pos)) { printf("Bit at position %d is set to 1.\n", pos); } else { printf("Bit at position %d is not set to 1.\n", pos); } return 0; }
How it works
- The program prompts the user to enter an integer number.
- The user enters a number, and it is stored in the variable
num
. - The program prompts the user to enter the bit position to check.
- The user enters a bit position, and it is stored in the variable
pos
. - The program checks if the
pos
value is within the valid range of 0 to 31. If it is not, an error message is displayed, and the program terminates. - If the
pos
value is valid, the program proceeds to theisBitSet
function. - The
isBitSet
function takes thenum
andpos
values as parameters. - Inside the
isBitSet
function, a mask is created by left-shifting 1 by thepos
value. This creates a binary number where only the bit at positionpos
is set to one, and all other bits are zero. - The
num
value is bitwise ANDed with the mask using the&
operator. - If the result of the bitwise AND operation is non-zero, it means the bit at position
pos
innum
is set to one. In this case, the function returns 1. - If the result of the bitwise AND operation is zero, it means the bit at position
pos
innum
is not set to one. In this case, the function returns 0. - Back in the
main
function, the program checks the return value of theisBitSet
function. - If the return value is 1, the program displays a message indicating that the bit is set to one.
- If the return value is 0, the program displays a message indicating that the bit is not set to one.
- The program terminates.
By following these steps, the program allows the user to input a number and a bit position, and it determines whether the bit at that position in the binary representation of the number is set to one or not.
Input/Output
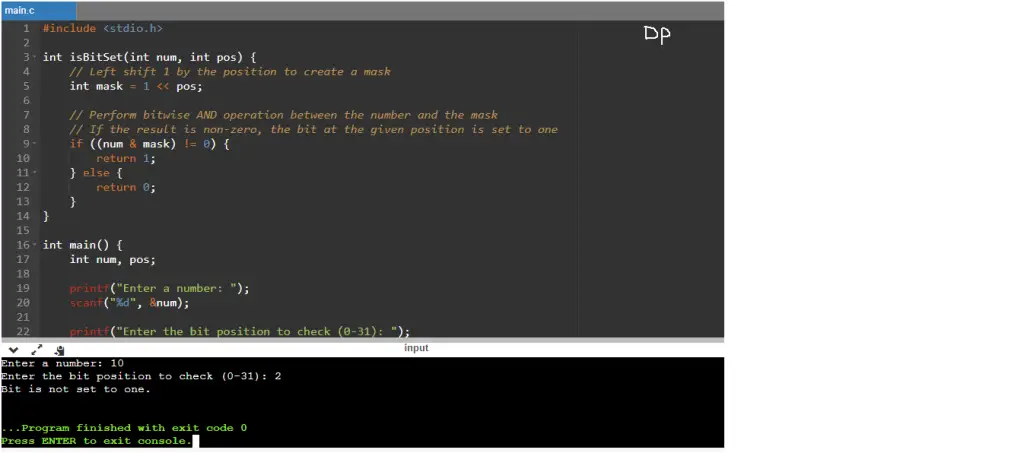