This C program performs matrix multiplication. Matrix multiplication is a fundamental operation in linear algebra, where two matrices are multiplied to produce a third matrix.
Matrix multiplication is a fundamental operation in linear algebra and has various applications in fields such as computer graphics, physics simulations, and optimization algorithms. In the context of computer programming, matrix multiplication involves multiplying two matrices to obtain a resultant matrix.
Program Statement
Write a C program to perform matrix multiplication of two input matrices and display the resultant matrix. The program should define a function matrixMultiplication
that takes two input matrices mat1
and mat2
as parameters and computes the resultant matrix res
. The program should also include a function displayMatrix
to display the contents of a matrix on the console.
C Program to Perform Matrix Multiplication
#include <stdio.h> #define ROW_A 3 #define COL_A 2 #define ROW_B 2 #define COL_B 3 void multiplyMatrices(int matA[][COL_A], int matB[][COL_B], int result[][COL_B]) { int i, j, k; for (i = 0; i < ROW_A; i++) { for (j = 0; j < COL_B; j++) { result[i][j] = 0; for (k = 0; k < COL_A; k++) { result[i][j] += matA[i][k] * matB[k][j]; } } } } void displayMatrix(int matrix[][COL_B], int row, int col) { int i, j; for (i = 0; i < row; i++) { for (j = 0; j < col; j++) { printf("%d\t", matrix[i][j]); } printf("\n"); } } int main() { int matrixA[ROW_A][COL_A] = {{1, 2}, {3, 4}, {5, 6}}; int matrixB[ROW_B][COL_B] = {{7, 8, 9}, {10, 11, 12}}; int resultMatrix[ROW_A][COL_B]; multiplyMatrices(matrixA, matrixB, resultMatrix); printf("Matrix A:\n"); displayMatrix(matrixA, ROW_A, COL_A); printf("Matrix B:\n"); displayMatrix(matrixB, ROW_B, COL_B); printf("Resultant Matrix:\n"); displayMatrix(resultMatrix, ROW_A, COL_B); return 0; }
How it Works
Here’s a step-by-step explanation of how the provided C program works to perform matrix multiplication:
- The program defines the dimensions of the matrices using constants
ROWS
andCOLS
. In this example, both matrices are 3×3, but you can adjust the dimensions as needed. - The
matrixMultiplication
function takes three parameters:mat1
,mat2
, andres
. These parameters represent the two input matrices and the resultant matrix, respectively. - Inside the
matrixMultiplication
function, three nested loops are used to iterate over the rows and columns of the matrices. The outer loop iterates over the rows ofmat1
, the middle loop iterates over the columns ofmat2
, and the inner loop calculates the dot product of the corresponding row and column. - For each element in the resultant matrix
res[i][j]
, the inner loop calculates its value by multiplying the corresponding elements frommat1
andmat2
and accumulating the results. The accumulation is performed by adding the product to the existing value ofres[i][j]
. - The
displayMatrix
function is defined to display the contents of a matrix on the console. It takes a matrixmat
as a parameter and uses nested loops to iterate over its rows and columns. The elements of the matrix are printed row by row usingprintf
. - In the
main
function, two input matricesmat1
andmat2
are defined and initialized with appropriate values. The resultant matrixresult
is also defined. - The
matrixMultiplication
function is called withmat1
,mat2
, andresult
as arguments to compute the resultant matrix. - After the matrix multiplication is performed, the program displays the input matrices
mat1
andmat2
using thedisplayMatrix
function. - Finally, the program displays the resultant matrix
result
using thedisplayMatrix
function.
By executing these steps, the program performs matrix multiplication and displays the input matrices as well as the resultant matrix on the console, allowing you to observe the outcome of the matrix multiplication operation.
Input/Output
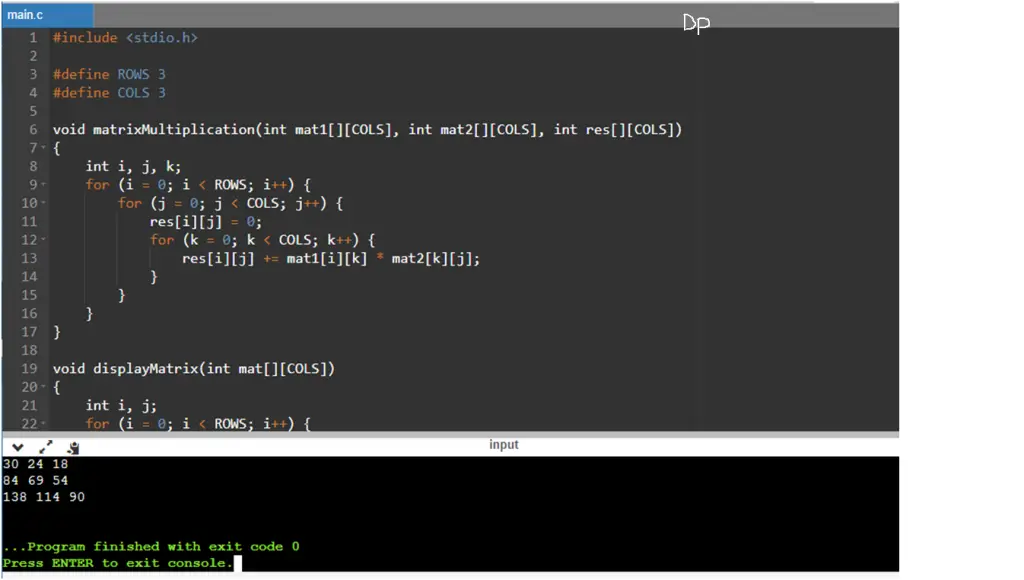