Intro
This C program demonstrates how to delete an element from an array. It prompts the user to enter an element to be deleted and performs the deletion operation. If the element is found in the array, it is removed, and the updated array is displayed. If the element is not present, a message indicating its absence is shown.
Problem statement
Write a C program to delete an element from an array. The program should take an array and its size as input from the user. Then it should prompt the user to enter the element to be deleted. If the element is found in the array, it should be removed, and the updated array should be displayed. If the element is not present, a message indicating its absence should be shown.
C program to Delete an Element from an Array
#include <stdio.h> void deleteElement(int arr[], int size, int element) { int i, j; // Find the element in the array for (i = 0; i < size; i++) { if (arr[i] == element) { // Shift elements to the left to overwrite the element for (j = i; j < size - 1; j++) { arr[j] = arr[j + 1]; } // Reduce the size of the array size--; break; } } if (i == size) { printf("Element not found in the array.\n"); } else { printf("Element deleted successfully.\n"); printf("Updated array: "); for (i = 0; i < size; i++) { printf("%d ", arr[i]); } printf("\n"); } } int main() { int arr[] = {1, 2, 3, 4, 5}; int size = sizeof(arr) / sizeof(arr[0]); int element; printf("Enter the element to delete: "); scanf("%d", &element); deleteElement(arr, size, element); return 0; }
How it works
- The program defines a function
deleteElement
that takes an array, its size, and the element to be deleted as parameters. - It iterates through the array to find the specified element.
- If the element is found, it shifts the subsequent elements to the left to overwrite the element and reduces the size of the array.
- If the element is not found, it prints a message indicating the element was not found.
- In the
main
function, an array is initialized, and the size of the array is calculated. - The user is prompted to enter the element to delete.
- The
deleteElement
function is called with the array, size, and element as arguments. - The function performs the deletion and displays the updated array if the element is found.
Input / output
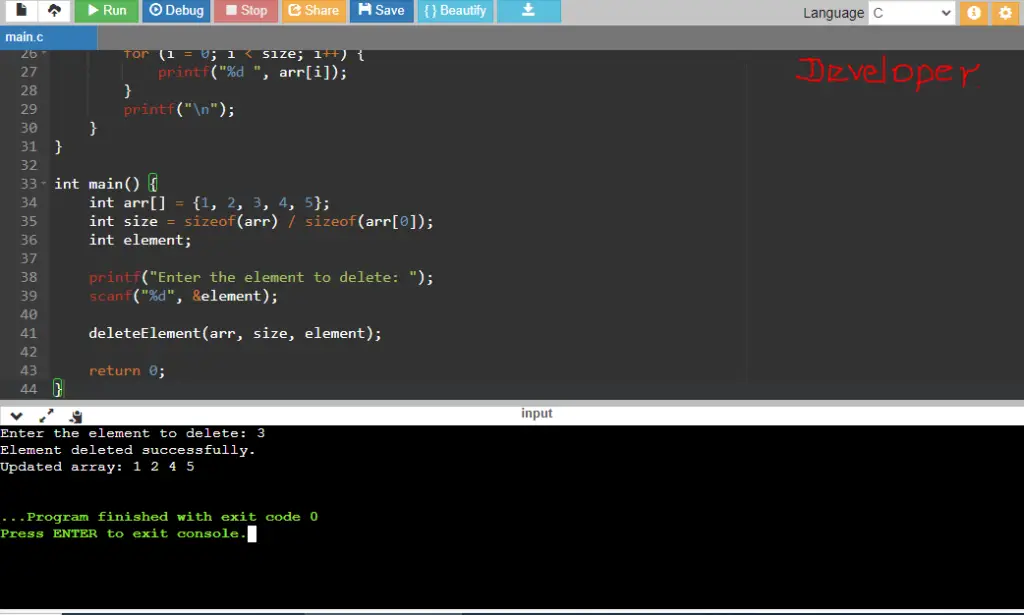