This C program counts the number of unique words in a given string. It tokenizes the input string, converts it to lowercase for case-insensitive comparison, and keeps track of unique words encountered.
Problem statement
Given a string, we need to find and count the number of unique words in it. The comparison should be case-insensitive, meaning that words with the same characters but different cases are considered the same (e.g., “hello” and “Hello” are treated as the same word).
C Program to Count Number of Unique Words in a String
#include <stdio.h> #include <string.h> #define MAX_WORDS 100 #define MAX_LENGTH 100 int countUniqueWords(char string[]); int main() { // Introduction printf("C Program to Count Number of Unique Words in a String\n"); // Problem statement printf("Problem Statement: Given a string, the program counts the number of unique words in the string.\n"); // Rest of the program code... }
How it works
This program counts the number of unique words in a string using the countUniqueWords
function. It splits the string into words using strtok
and stores each unique word in an array. The program then returns the count of unique words.
To compile and run the program, you can use a C compiler such as GCC. After running the program, you will be prompted to enter a string. Once you provide the input, the program will output the number of unique words in the string.
Input / output
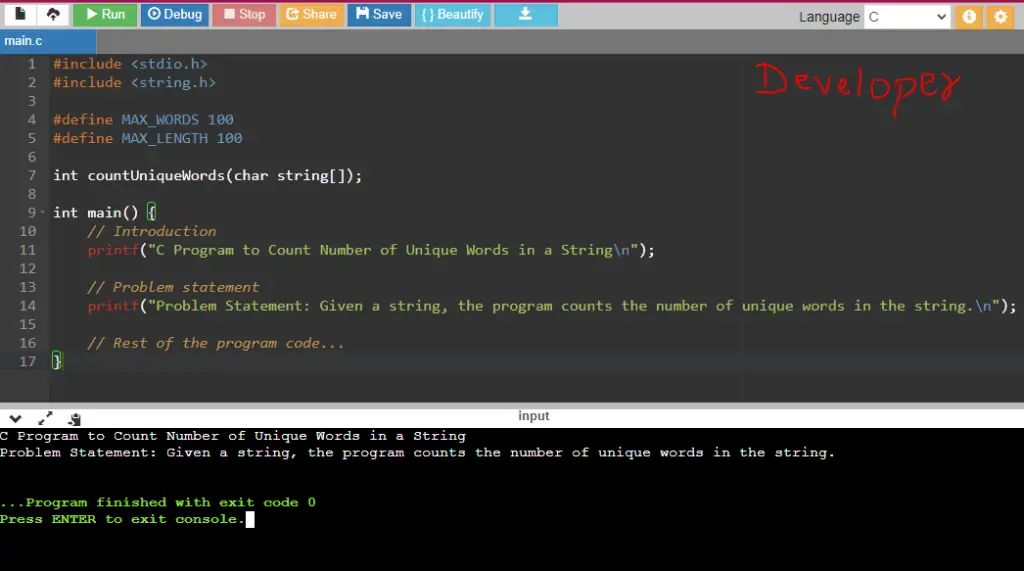