This C program calculates and displays the position of the highest bit set (most significant bit) for a given integer. The highest bit set is the leftmost (most significant) bit that is turned on (1) in the binary representation of the integer.
Problem Statement
Write a C program that finds the position of the highest bit set (most significant bit) for a given integer.
C Program to Find the Highest Bit Set for any Given Integer
#include <stdio.h> int findHighestBitSet(int num) { int position = 0; while (num > 0) { num = num >> 1; // Right shift by 1 bit position++; } return position; } int main() { int num; printf("Enter an integer: "); scanf("%d", &num); int highestBit = findHighestBitSet(num); printf("The highest bit set in %d is at position %d.\n", num, highestBit); return 0; }
How it works
Let’s go through how the program works step by step:
- The program starts by including the necessary header file
stdio.h
for input/output operations. - The
findHighestBitSet
function is defined. It takes an integernum
as input and returns the position of the highest bit set. - Inside the
findHighestBitSet
function, an integer variableposition
is declared and initialized to 0. This variable will keep track of the bit position. - The program enters a
while
loop that continues untilnum
becomes 0. This loop is responsible for right-shiftingnum
by 1 bit in each iteration. - Inside the loop,
num
is right-shifted by 1 bit using the>>=
operator. This operation effectively dividesnum
by 2. - The
position
variable is incremented by 1 in each iteration to keep track of the number of right shifts performed. - Once
num
becomes 0, the loop exits, and the value ofposition
represents the position of the highest bit set in the original number. - The
main
function is defined. It is the entry point of the program. - Inside
main
, an integer variablenum
is declared to store the user input. - The user is prompted to enter an integer using the
printf
function. - The
scanf
function is used to read the user input and store it in thenum
variable. - The
findHighestBitSet
function is called with thenum
variable as an argument. The returned value, representing the position of the highest bit set, is stored in thehighestBitPosition
variable. - Finally, the program outputs the highest bit set position using the
printf
function.
That’s how the program works! It takes an input integer, finds the position of the highest bit set using a right-shift operation, and displays the result to the user.
Input/Output
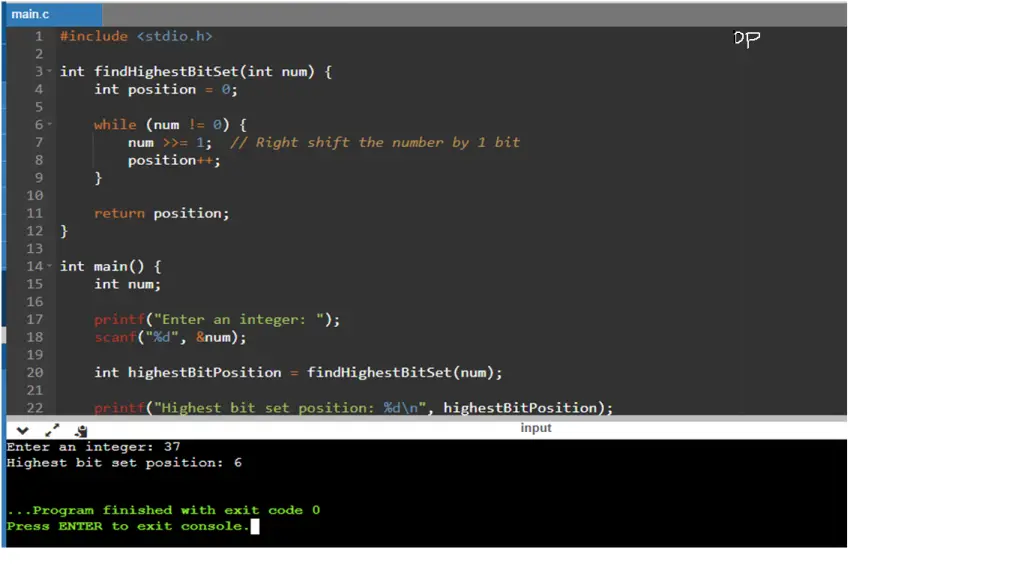