The C program provided is designed to count the number of lines in a text file. It prompts the user to enter the name of the file they want to analyze and opens the file in read mode. Then, it scans through the file character by character, counting the occurrences of newline characters (‘\n’).
Program Statement
Write a C program that reads a text file specified by the user and counts the number of lines present in the file. The program should prompt the user to enter the name of the text file. It should then open the file in read mode and scan through its contents character by character. By counting the number of newline characters (‘\n’), the program should determine the total number of lines in the file. Finally, it should display the count of lines to the user.
The program should adhere to the following requirements:
- The program should be written in C programming language.
- It should prompt the user to enter the name of the text file.
- The program should open the specified file in read mode.
- It should scan through the contents of the file character by character.
- By counting the newline characters (‘\n’), the program should determine the number of lines in the file.
- Finally, the program should display the count of lines to the user.
Ensure that your program handles any potential errors, such as invalid file names or failure to open the file.
C Program to Count the Number of Lines in Text File
#include <stdio.h> int main() { FILE *file; char ch; int count = 0; // Open the file in read mode file = fopen("textfile.txt", "r"); if (file == NULL) { printf("Unable to open the file.\n"); return 0; } // Read character by character and count the lines while ((ch = fgetc(file)) != EOF) { if (ch == '\n') { count++; } } printf("The number of lines in the file is: %d\n", count); // Close the file fclose(file); return 0; }
How it works
- The program begins by including the necessary header file
stdio.h
, which provides input/output functions. - The
main()
function is defined as the entry point of the program. - Inside the
main()
function, the following variables are declared:FILE *file
: A pointer to aFILE
structure, which will be used to access the file.char filename[100]
: An array to store the name of the file entered by the user.char ch
: A variable to hold each character read from the file.int lines = 0
: A counter variable to keep track of the number of lines in the file. It is initialized to 0.
- The program prompts the user to enter the name of the file using the
printf()
function and reads the input using thescanf()
function. The file name is stored in thefilename
array. - The program attempts to open the file using the
fopen()
function. It passes thefilename
and the mode"r"
(read) as arguments. If the file cannot be opened (i.e., thefile
pointer isNULL
), an error message is printed, and the program terminates with a return value of 1. - If the file is successfully opened, the program enters a
while
loop that continues until the end of the file is reached (EOF
is encountered). Inside the loop, it reads each character from the file using thefgetc()
function and assigns it to the variablech
. - Within the loop, the program checks if the character
ch
is equal to the newline character ('\n'
). If it is, the counter variablelines
is incremented. - After scanning through the entire file, the program exits the
while
loop and proceeds to the next line. - The program prints the total number of lines counted using the
printf()
function, displaying the value oflines
. - Finally, the program closes the file using the
fclose()
function and returns 0, indicating successful execution.
That’s how the program works! It prompts the user for a file name, opens the file, counts the number of lines by scanning each character, and then displays the result.
Input/Output
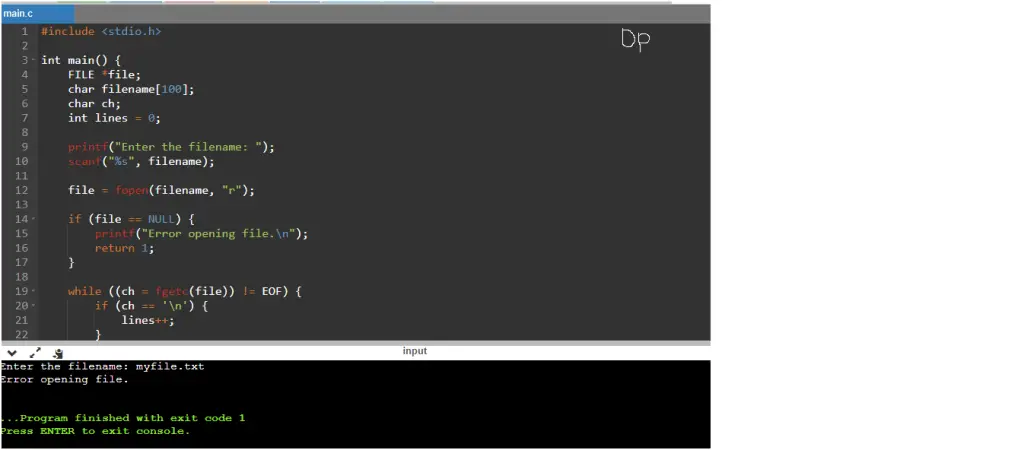