Floyd’s Triangle is a triangular number pattern named after Robert Floyd. It is a right-angled triangle where the numbers are filled row by row. In this C program, we generate Floyd’s Triangle based on the user’s input for the number of rows.
Problem Statement
Given a positive integer ‘rows’, this program prints Floyd’s Triangle with ‘rows’ number of rows.
The program prompts the user to enter the number of rows they want in the Floyd’s Triangle. Then, it generates and prints the triangle using nested loops. Each row contains the numbers from 1 to ‘rows’ (incremented by 1 each time). Finally, the program terminates.
C Program to Print Floyd’s Triangle
#include <stdio.h> int main() { int rows, i, j, num = 1; printf("Floyd's Triangle\n\n"); printf("Enter the number of rows: "); scanf("%d", &rows); printf("\n"); for (i = 1; i <= rows; i++) { for (j = 1; j <= i; j++) { printf("%d ", num); num++; } printf("\n"); } return 0; }
How it works
- We start by including the necessary header file
stdio.h
for standard input/output operations. - In the
main
function, we declare variablesrows
(to store the number of rows),i
,j
(loop counters), andnum
(to keep track of the numbers to be printed). - We prompt the user to enter the number of rows they want in the Floyd’s Triangle.
- Using a nested
for
loop, we iterate over each row and column to print the numbers in the triangle. - The outer loop
i
controls the number of rows, and the inner loopj
prints the numbers in each row. - Inside the inner loop, we print the value of
num
followed by a space, and then incrementnum
by 1. - After printing each row, we move to the next line using
printf("\n")
. - Once all the rows are printed, the program ends.
Input / Output
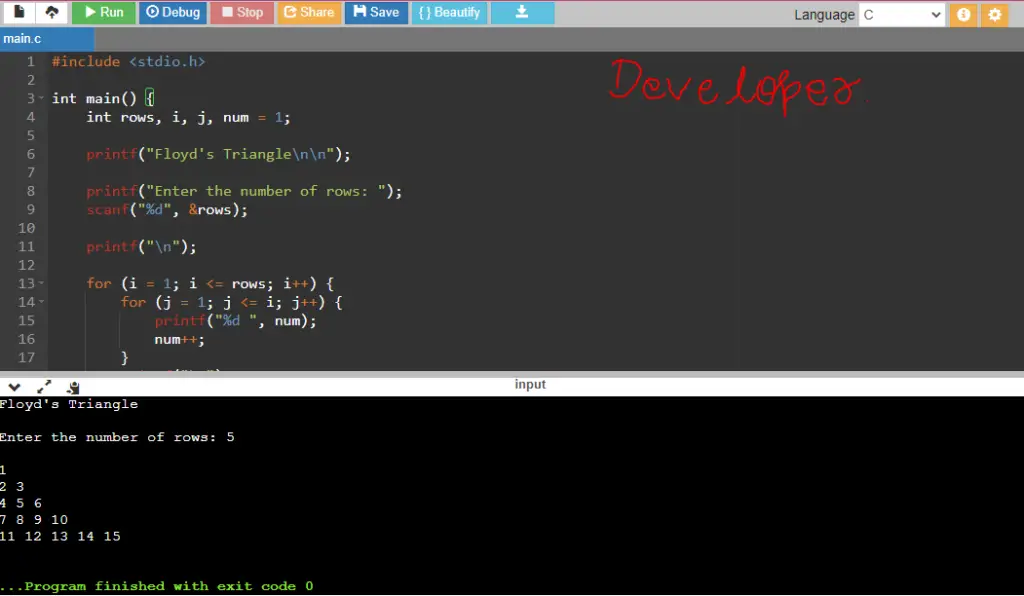