This C program converts a hexadecimal number to its binary representation. It takes a hexadecimal number as input and produces the corresponding binary representation as output.
Problem Statement
Write a C program to convert a hexadecimal number to binary.
C Program to Convert Hexadecimal Number to Binary
#include <stdio.h> // Function to convert hexadecimal to binary void hexToBinary(char hex[]) { printf("Hexadecimal number: %s\n", hex); printf("Binary number: "); int i = 0; while (hex[i]) { switch (hex[i]) { case '0': printf("0000"); break; case '1': printf("0001"); break; case '2': printf("0010"); break; case '3': printf("0011"); break; case '4': printf("0100"); break; case '5': printf("0101"); break; case '6': printf("0110"); break; case '7': printf("0111"); break; case '8': printf("1000"); break; case '9': printf("1001"); break; case 'A': case 'a': printf("1010"); break; case 'B': case 'b': printf("1011"); break; case 'C': case 'c': printf("1100"); break; case 'D': case 'd': printf("1101"); break; case 'E': case 'e': printf("1110"); break; case 'F': case 'f': printf("1111"); break; default: printf("\nInvalid hexadecimal digit %c", hex[i]); return; } i++; } } int main() { char hex[20]; printf("Enter a hexadecimal number: "); scanf("%s", hex); hexToBinary(hex); return 0; }
How it works
- The program prompts the user to enter a hexadecimal number.
- The
hexToBinary
function is called with the entered hexadecimal number as an argument. - Inside the
hexToBinary
function, a loop is used to iterate through each character of the hexadecimal number. - Using a switch case, each hexadecimal digit is converted to its binary representation.
- The binary representation is printed to the console.
Input / Output
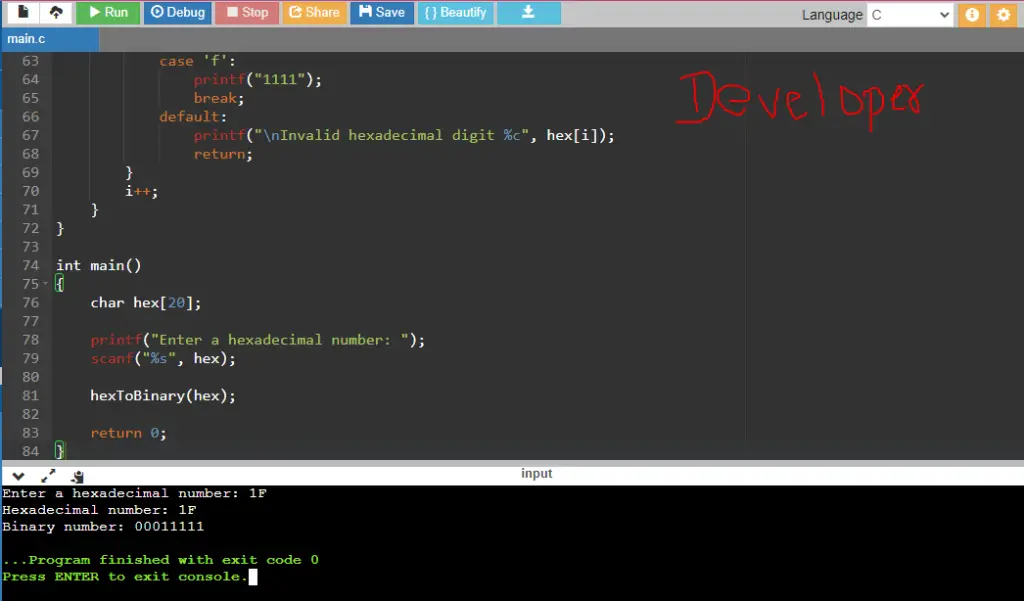