This C program converts a binary number to its hexadecimal equivalent.
Problem Statement
Write a program that takes a binary number as input and converts it to its hexadecimal representation.
C Program to Convert Binary to Hexadecimal
#include <stdio.h> #include <string.h> // Function to convert binary to hexadecimal void binaryToHexadecimal(char binary[]) { // Length of the binary number int length = strlen(binary); // Calculating the number of digits required in hexadecimal int hexLength = (length + 3) / 4; // Padding the binary number with leading zeros if necessary int paddingZeros = hexLength * 4 - length; for (int i = 0; i < paddingZeros; i++) { printf("0"); } // Converting each group of 4 binary digits to hexadecimal for (int i = 0; i < length; i += 4) { int decimal = 0; // Converting binary to decimal for (int j = 0; j < 4; j++) { decimal = decimal * 2 + (binary[i + j] - '0'); } // Converting decimal to hexadecimal if (decimal < 10) { printf("%c", decimal + '0'); } else { printf("%c", decimal - 10 + 'A'); } } printf("\n"); } int main() { char binary[100]; printf("Binary to Hexadecimal Converter\n"); printf("-------------------------------\n"); printf("Enter a binary number: "); scanf("%s", binary); printf("Hexadecimal equivalent: "); binaryToHexadecimal(binary); return 0; }
How it works
- The program defines a function called
binaryToHexadecimal
that takes a binary number as input and converts it to its hexadecimal equivalent. - The function calculates the number of hexadecimal digits required based on the length of the binary number.
- It then pads the binary number with leading zeros if necessary to form complete groups of 4 digits.
- The function processes each group of 4 binary digits and converts them to decimal.
- Finally, it converts the decimal value to its corresponding hexadecimal representation and prints the result.
Input / Output
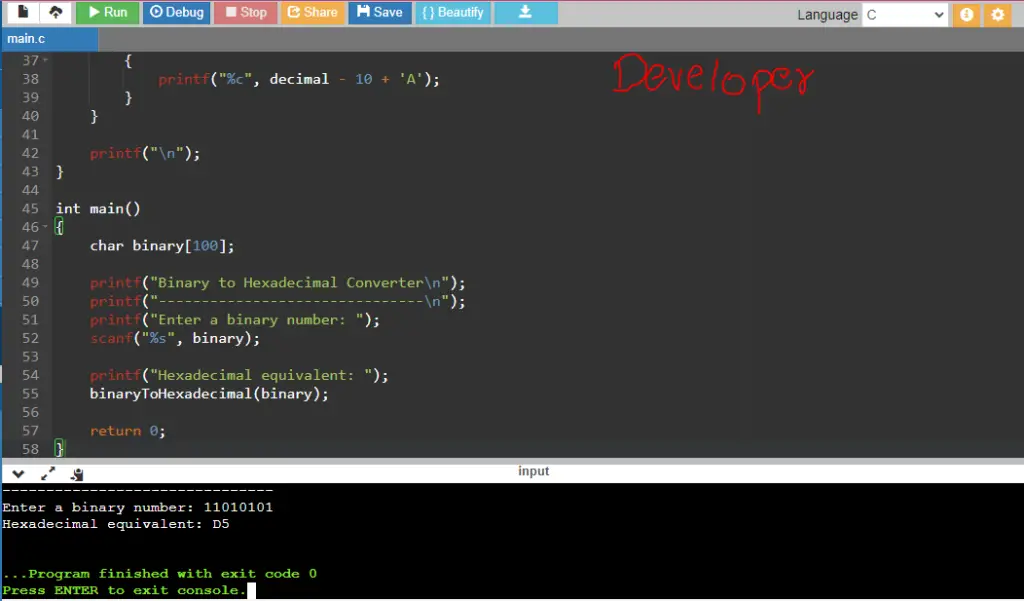