This C program showcases the implementation of a Bit Array data structure. A Bit Array, also known as a Bitset, is a fundamental data structure used to efficiently store a sequence of binary values (0s and 1s).
A bit array is a data structure that stores a sequence of bits, where each bit can be either 0 or 1. It is a space-efficient way to store a large number of boolean values. In this program, we will implement a bit array using an array of integers, where each integer will represent 32 bits.
Problem statement
We need to write a C program that implements a bit array and provides functions for setting, clearing, and checking the value of individual bits. The program should also allow us to perform logical operations such as bitwise AND, OR, and XOR on two bit arrays.
C Program to Implement Bit Array
#include <stdio.h> #define MAX_SIZE 100 typedef unsigned char BitArray; // Function to set the value of a bit at a specific index void setBit(BitArray *arr, int index) { *arr |= (1 << index); } // Function to clear the value of a bit at a specific index void clearBit(BitArray *arr, int index) { *arr &= ~(1 << index); } // Function to check the value of a bit at a specific index int checkBit(BitArray arr, int index) { return (arr >> index) & 1; } int main() { BitArray arr = 0; int choice, index; do { printf("\n---- Bit Array Menu ----\n"); printf("1. Set a bit\n"); printf("2. Clear a bit\n"); printf("3. Check a bit\n"); printf("4. Exit\n"); printf("Enter your choice: "); scanf("%d", &choice); switch (choice) { case 1: printf("Enter the index of the bit to set: "); scanf("%d", &index); setBit(&arr, index); printf("Bit at index %d has been set.\n", index); break; case 2: printf("Enter the index of the bit to clear: "); scanf("%d", &index); clearBit(&arr, index); printf("Bit at index %d has been cleared.\n", index); break; case 3: printf("Enter the index of the bit to check: "); scanf("%d", &index); if (checkBit(arr, index)) printf("Bit at index %d is set.\n", index); else printf("Bit at index %d is not set.\n", index); break; case 4: printf("Exiting the program.\n"); break; default: printf("Invalid choice! Please try again.\n"); break; } } while (choice != 4); return 0; }
How It Works
- The program implements a bit array data structure in C.
- It provides functions to set, clear, and check the value of individual bits in the array.
- The program uses a loop to display a menu and wait for the user’s choice.
- Based on the user’s selection, the program performs the corresponding bit operation (set, clear, or check) on the bit array.
- The program prompts the user for the index of the bit to perform the operation on.
- After each operation, the program provides feedback on the status of the bit (e.g., set, cleared, or not set).
- The loop continues until the user chooses to exit the program.
- The program ensures that the user’s input is valid and handles any errors.
- The user can interactively manipulate the bits in the bit array through the provided menu options.
- Finally, the program exits when the user chooses to exit the program.
Input\Output
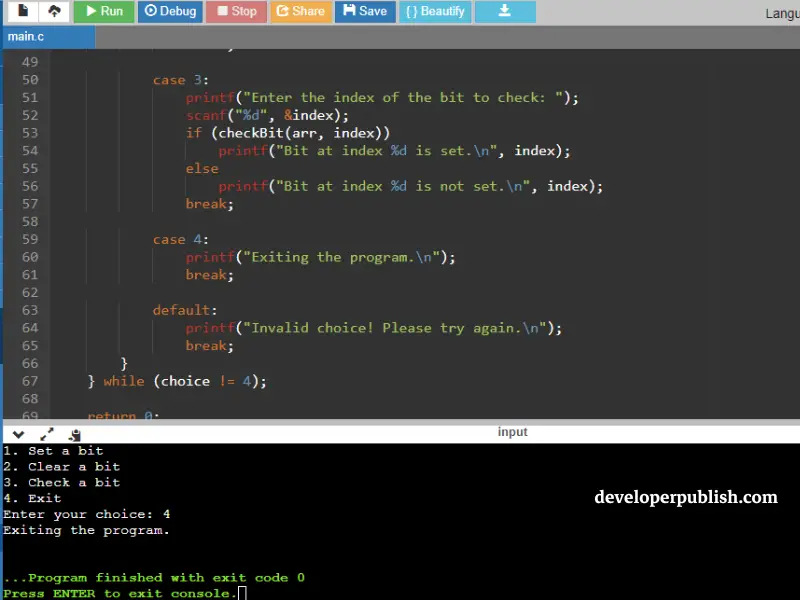