This C program is designed to count the number of trailing zeros in the binary representation of an integer. Trailing zeros are zeros that appear at the end (least significant bit) of a binary number. This program provides a simple and efficient way to count trailing zeros in an integer’s binary representation.
Problem statement
Problem: Count Trailing Zeros in an Integer Write a program that takes an integer as input and counts the number of trailing zeros in its binary representation. A trailing zero is defined as a zero bit at the end (least significant bit) of the binary representation.
Your program should perform the following steps:
- Prompt the user to enter an integer.
- Read the integer from the user.
- Convert the integer to its binary representation.
- Count the number of trailing zeros in the binary representation.
- Display the count of trailing zeros as output
C Program to Count Trailing Zeros in Integer
#include <stdio.h> int countTrailingZeros(int n) { int count = 0; // Loop until the rightmost bit is 1 while ((n & 1) == 0) { count++; n >>= 1; // Right shift by 1 bit } return count; } int main() { int number; printf("Enter an integer: "); scanf("%d", &number); int zeros = countTrailingZeros(number); printf("Number of trailing zeros: %d\n", zeros); return 0; }
How it works
- The program prompts the user to enter an integer.
- The entered integer is read from the user and stored in the variable
number
. - The program calls the function
countTrailingZeros
with thenumber
as an argument. - Inside the
countTrailingZeros
function, the variablecount
is initialized to 0. This variable will keep track of the number of trailing zeros. - The while loop
(n & 1) == 0
checks if the rightmost bit ofn
is 0. If it is, it means there is a trailing zero. - Inside the loop, the
count
variable is incremented by 1, andn
is right-shifted by 1 bit using the>>=
operator. This shift effectively removes the rightmost bit. - The loop continues until the rightmost bit of
n
becomes 1, indicating the end of trailing zeros. - Finally, the function returns the value of
count
. - Back in the
main
function, the returned value fromcountTrailingZeros
is stored in the variablezeros
. - The program displays the number of trailing zeros by printing the value of
zeros
using theprintf
function.
By following these steps, the program correctly counts the number of trailing zeros in the binary representation of the entered integer and displays the result
Input / Output
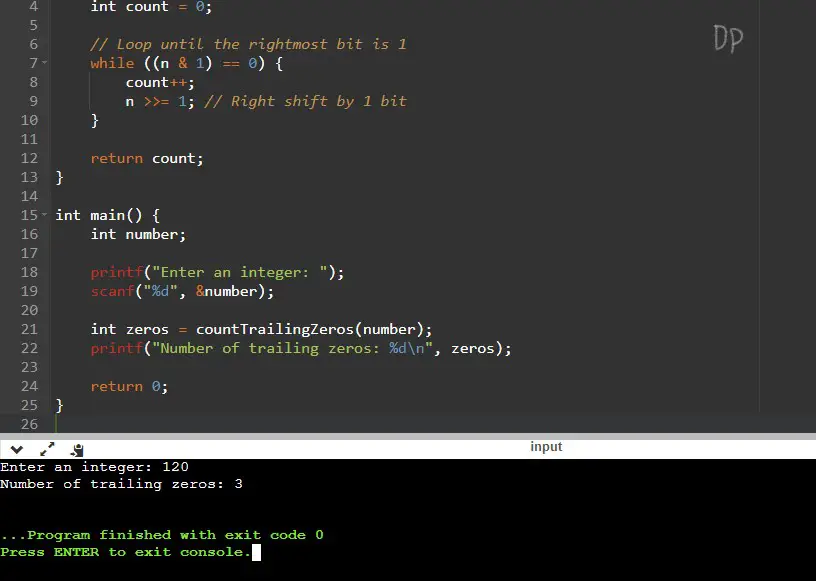