This C program checks whether a given string is a palindrome or not without using any built-in string reversal function.
Problem Statement
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward, disregarding spaces, punctuation, and capitalization.
Requirements:
- The program should prompt the user to enter a string.
- It should then determine whether the entered string is a palindrome.
- The program should perform the palindrome check without using any built-in functions.
- The comparison should be case-sensitive, meaning uppercase and lowercase letters should be treated as distinct.
- The program should output an appropriate message indicating whether the entered string is a palindrome or not.
C Program to Check if a String is a Palindrome without using Built-in Function
#include <stdio.h> // Function to check if a string is a palindrome int isPalindrome(char *str) { int length = 0; int i, j; // Find the length of the string while (str[length] != '\0') { length++; } // Check if the string is a palindrome for (i = 0, j = length - 1; i < j; i++, j--) { if (str[i] != str[j]) { return 0; // Not a palindrome } } return 1; // Palindrome } int main() { char str[100]; printf("Enter a string: "); scanf("%s", str); if (isPalindrome(str)) { printf("%s is a palindrome.\n", str); } else { printf("%s is not a palindrome.\n", str); } return 0; }
How it Works
- The program prompts the user to enter a string.
- The entered string is stored in an array in the
main
function. - The
isPalindrome
function is called with the entered string as an argument. - Inside the
isPalindrome
function:- The length of the string is calculated by iterating through it until the null character (
'\0'
) is encountered. - Two variables,
i
andj
, are initialized to keep track of the start and end indices of the string for comparison. - A loop is executed from the start and end of the string towards the middle.
- During each iteration, the characters at indices
i
andj
are compared.- If they are different, the function returns
0
to indicate that the string is not a palindrome. - If they are the same, the loop continues to the next iteration.
- If they are different, the function returns
- If the loop completes without finding any differences, the function returns
1
to indicate that the string is a palindrome.
- The length of the string is calculated by iterating through it until the null character (
- The return value of the
isPalindrome
function is used in themain
function to determine whether the entered string is a palindrome or not. - Based on the return value, the appropriate message is printed to the console indicating whether the string is a palindrome or not.
The program performs the palindrome check by comparing characters at symmetric positions in the string, starting from both ends and moving towards the middle. If any pair of characters is found to be different, the string is determined to be not a palindrome. Conversely, if all the pairs of characters match, the string is determined to be a palindrome.
Input /Output
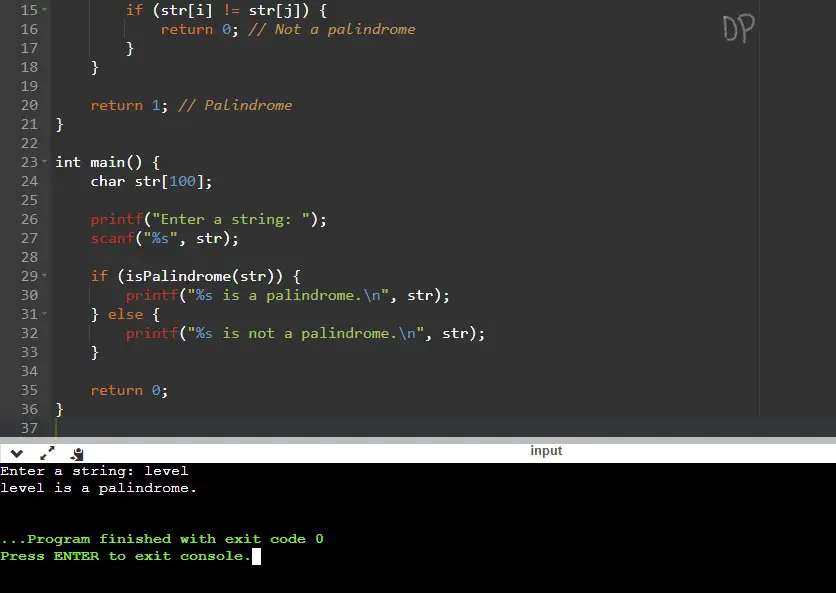
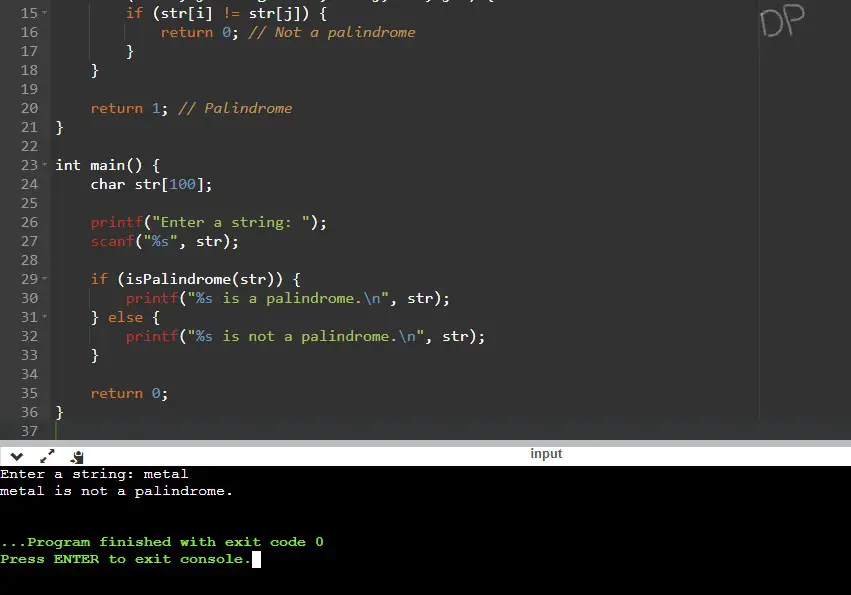