This C program finds the largest and smallest possible words, which are palindromes, from a given input sentence.
Problem Statement
Write a C program that prompts the user to enter a sentence and finds the largest and smallest possible words that are palindromes within the sentence. The program should consider the case-insensitivity of palindromes, treating uppercase and lowercase letters as the same.
A palindrome is a word, phrase, number, or sequence of characters that reads the same forward and backward.
The program should perform the following steps:
- Prompt the user to enter a sentence.
- Read the input sentence.
- Process the input sentence and identify palindromic words.
- Consider each word in the sentence by iterating through the characters.
- Convert the word to lowercase for case-insensitive comparison.
- Determine if the word is a palindrome by comparing the characters from the beginning and end.
- Update the largestPalindrome and smallestPalindrome variables accordingly when a palindrome is found.
- After processing the entire sentence, output the largest and smallest palindromic words found.
- Handle words separated by spaces and consider them as separate entities.
Assumptions:
- The maximum length of a word is 100 characters.
- The maximum length of the input sentence is 100 characters.
Note: You can adjust these assumptions and constants in the program based on your requirements.
C Program to Find the Largest & Smallest possible Word which is a Palindrome
#include <stdio.h> #include <string.h> #include <ctype.h> #define MAX_LENGTH 100 int isPalindrome(char *word) { int length = strlen(word); int i, j; // Convert word to lowercase for case-insensitive comparison for (i = 0; i < length; i++) { word[i] = tolower(word[i]); } // Check if word is a palindrome for (i = 0, j = length - 1; i < j; i++, j--) { if (word[i] != word[j]) { return 0; } } return 1; } void findPalindromes(char *sentence) { char word[MAX_LENGTH]; char largestPalindrome[MAX_LENGTH] = ""; char smallestPalindrome[MAX_LENGTH] = ""; int length = strlen(sentence); int i, j, k = 0; for (i = 0; i <= length; i++) { if (sentence[i] != ' ' && sentence[i] != '\0') { word[k++] = sentence[i]; } else { word[k] = '\0'; if (k > 0 && isPalindrome(word)) { if (strlen(word) > strlen(largestPalindrome)) { strcpy(largestPalindrome, word); } if (strlen(word) < strlen(smallestPalindrome) || strlen(smallestPalindrome) == 0) { strcpy(smallestPalindrome, word); } } k = 0; } } printf("Largest palindrome: %s\n", largestPalindrome); printf("Smallest palindrome: %s\n", smallestPalindrome); } int main() { char sentence[MAX_LENGTH]; printf("Enter a sentence: "); fgets(sentence, sizeof(sentence), stdin); // Remove newline character from the end of the input sentence[strcspn(sentence, "\n")] = '\0'; findPalindromes(sentence); return 0; }
How it Works
- The program starts by prompting the user to enter a sentence.
- The input sentence is read and stored in a character array.
- The program defines a helper function called
isPalindrome
to check if a given word is a palindrome. This function takes a character array (word) as input and returns 1 if the word is a palindrome, and 0 otherwise. - The program defines another function called
findPalindromes
to process the input sentence and find the largest and smallest palindromic words. This function takes the input sentence as input. - Within the
findPalindromes
function, a character arrayword
is defined to temporarily store each word while processing the sentence. - The variables
largestPalindrome
andsmallestPalindrome
are initialized as empty character arrays. - The length of the sentence is calculated using the
strlen
function, and a loop is used to iterate through each character of the sentence. - Inside the loop, each character is checked to determine if it is a space or the end of the sentence.
- If a character is not a space or the end of the sentence, it is appended to the
word
array. - When a space or the end of the sentence is encountered, the
word
array is null-terminated, and theisPalindrome
function is called to check if the word is a palindrome. - If the word is a palindrome, it is compared with the existing
largestPalindrome
andsmallestPalindrome
. If the word is larger than thelargestPalindrome
or smaller than thesmallestPalindrome
, it is updated accordingly. - After processing all the words in the sentence, the
largestPalindrome
andsmallestPalindrome
are printed as the output. - Finally, the
main
function is executed. It prompts the user to enter a sentence, reads the input, and calls thefindPalindromes
to function to process the sentence and find the largest and smallest palindromic words.
The program uses the standard library functions strcpy
, strlen
, tolower
, and string comparison functions to perform various string operations and comparisons.
By executing this program with different input sentences, you can explore different examples of palindromic words and observe the program’s ability to identify the largest and smallest ones.
Input / Output
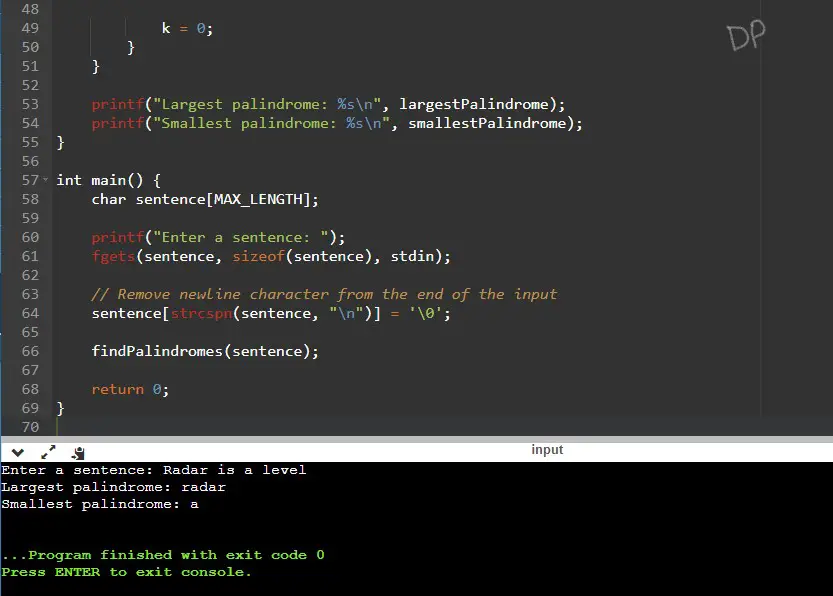
In this example, the input sentence is “Radar is a level”. The program identifies the largest palindrome as “radar” and the smallest palindrome as “a”. Note that the program ignores the case of the letters when determining palindromes, so it treats “Radar” and “radar” as the same word.