This C program calculates the area of a circle using its radius.
Problem Statement
- The program structure starts with the problem statement, followed by the input and output sections.
- The variable declaration, calculation, and formatting of the output remain the same as in the previous program.
C Program to Find the Area of a Circle
#include <stdio.h> int main() { // Intro printf("C Program to Calculate the Area of a Circle\n"); printf("------------------------------------------\n"); // Problem Statement printf("Given the radius of a circle, this program calculates its area.\n"); // Variables float radius, area; const float PI = 3.14159; // Input printf("\nEnter the radius of the circle: "); scanf("%f", &radius); // Calculation area = PI * radius * radius; // Output printf("\nThe area of the circle with radius %.2f is: %.2f\n", radius, area); return 0; }
How it works
- We begin by including the necessary header file,
stdio.h
, which provides input/output functionality. - Inside the
main
function, we declare the variablesradius
andarea
as floats, and the constantPI
with a value of 3.14159. - We display an introductory message and the problem statement using
printf
. - The user is prompted to enter the radius of the circle using
printf
. - The value entered by the user is read into the
radius
variable usingscanf
. - The area of the circle is calculated using the formula
area = PI * radius * radius
. - Finally, the calculated area is displayed on the screen using
printf
Input / output
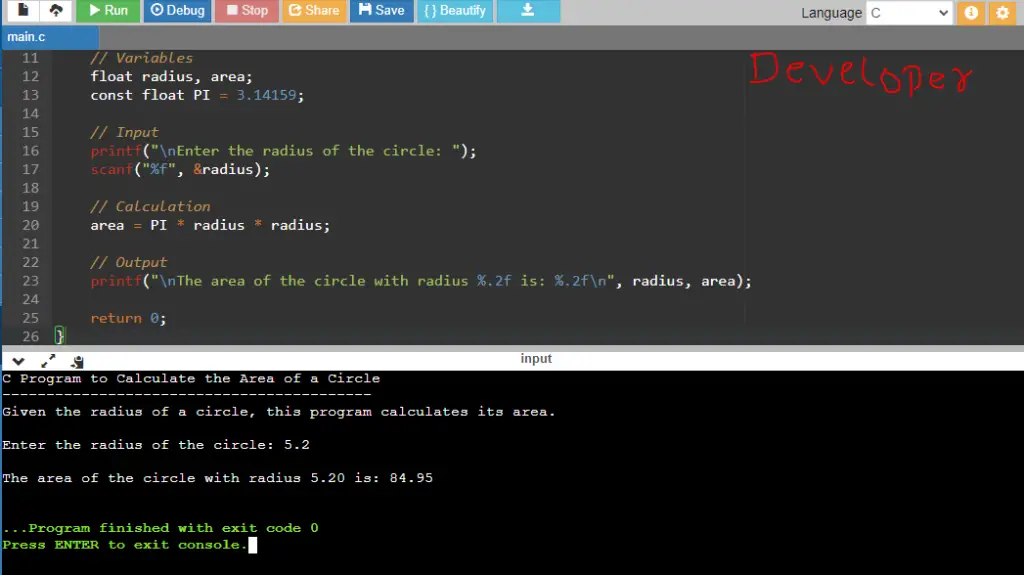