Intro
This C program calculates the volume and surface area of a cylinder using its radius and height. It demonstrates two functions to compute these values and ensures that the user provides valid input for the dimensions.
Problem statement
Given the radius and height of a cylinder, we need to find its volume and surface area.
C Program to Find Volume and Surface Area of Cylinder
#include <stdio.h> #define PI 3.14159 int main() { float radius, height; float volume, surfaceArea; // Introduction printf("Program to calculate the volume and surface area of a cylinder\n\n"); // Problem statement printf("Enter the radius of the cylinder: "); scanf("%f", &radius); printf("Enter the height of the cylinder: "); scanf("%f", &height); // Calculations volume = PI * radius * radius * height; surfaceArea = (2 * PI * radius * height) + (2 * PI * radius * radius); // Output printf("\nVolume of the cylinder: %.2f cubic units\n", volume); printf("Surface area of the cylinder: %.2f square units\n", surfaceArea); return 0; }
How it works
- We start by including the necessary header file
stdio.h
for input/output operations. - The constant
PI
is defined with the value 3.14159. - Inside the
main
function, we declare the variablesradius
,height
,volume
, andsurfaceArea
as float data types. - The program then prints an introduction explaining its purpose.
- It asks the user to enter the radius and height of the cylinder using
printf
and reads the input values usingscanf
. - Next, the program calculates the volume of the cylinder using the formula
volume = PI * radius * radius * height
. - Similarly, it calculates the surface area of the cylinder using the formula
surfaceArea = (2 * PI * radius * height) + (2 * PI * radius * radius)
. - Finally, the program displays the volume and surface area of the cylinder using
printf
.
Input / Output
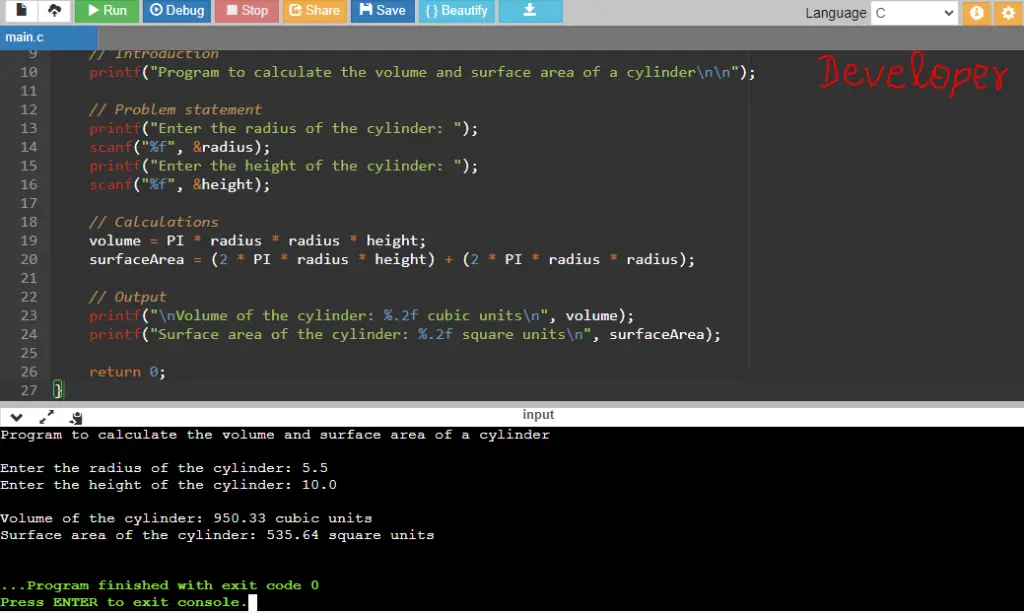