This C program shows how you can to Find Area of a Right Angled Triangle.
Problem statement
Write a C Program to Find Area of a Right Angled Triangle.
C Program to Find Area of a Right Angled Triangle
#include <stdio.h> int main() { float base, height, area; // Introduction printf("Program to calculate the area of a right-angled triangle.\n"); // Problem statement printf("Enter the base and height of the triangle:\n"); // User input printf("Base: "); scanf("%f", &base); printf("Height: "); scanf("%f", &height); // Calculate area area = 0.5 * base * height; // Output the result printf("The area of the right-angled triangle is: %f\n", area); return 0; }
How it works
- The program starts by declaring the necessary variables:
base
,height
, andarea
as floating-point numbers. - The introductory message is displayed using the
printf
function. - The problem statement asks the user to enter the base and height of the right-angled triangle.
- The program prompts the user for input using
printf
and reads the values of base and height using thescanf
function. - The area is calculated using the formula: area = 0.5 * base * height.
- The result is printed using the
printf
function. - Finally, the program ends and returns 0 to indicate successful execution.
Input / output
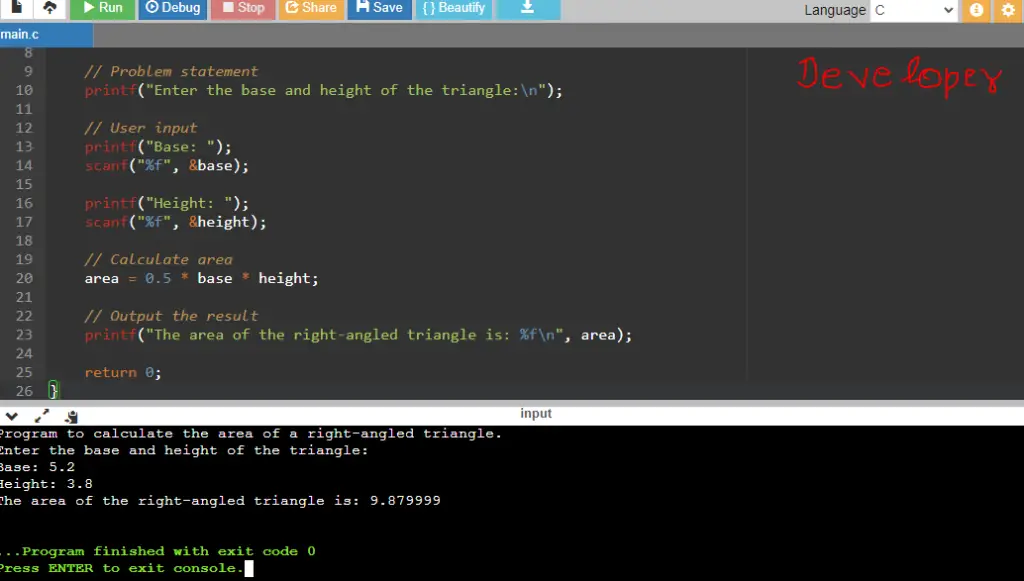