This C program determines whether a given year is a leap year or not. A leap year is a year that is exactly divisible by 4, except for years that are exactly divisible by 100. However, years that are divisible by 400 are leap years.
Problem statement
Write a C program to find if the given year is a leap year or not.
C Program to find if the Given Year is Leap Year or Not
#include <stdio.h> int isLeapYear(int year) { if (year % 400 == 0) { return 1; } else if (year % 100 == 0) { return 0; } else if (year % 4 == 0) { return 1; } else { return 0; } } int main() { int year; printf("Leap Year Checker\n"); printf("-----------------\n"); printf("Enter a year: "); scanf("%d", &year); if (isLeapYear(year)) { printf("%d is a leap year.\n", year); } else { printf("%d is not a leap year.\n", year); } return 0; }
How it works?
- The program starts by including the necessary header file
stdio.h
, which provides input/output functions. - The function
is LeapYear()
takes an integeryear
as input and returns 1 if the year is a leap year, and 0 if it’s not. - Inside the
is LeapYear()
function, it checks the following conditions:- If the year is divisible by 400, it is a leap year.
- If the year is divisible by 100, it is not a leap year.
- If the year is divisible by 4, it is a leap year.
- If none of the above conditions are met, it is not a leap year.
- In the
main()
function:- It prompts the user to enter a year.
- The input is stored in the
year
variable using thescanf()
function. - It calls the
isLeapYear()
function with theyear
as an argument to determine if it’s a leap year or not. - Finally, it prints the result based on the returned value.
Input/Output
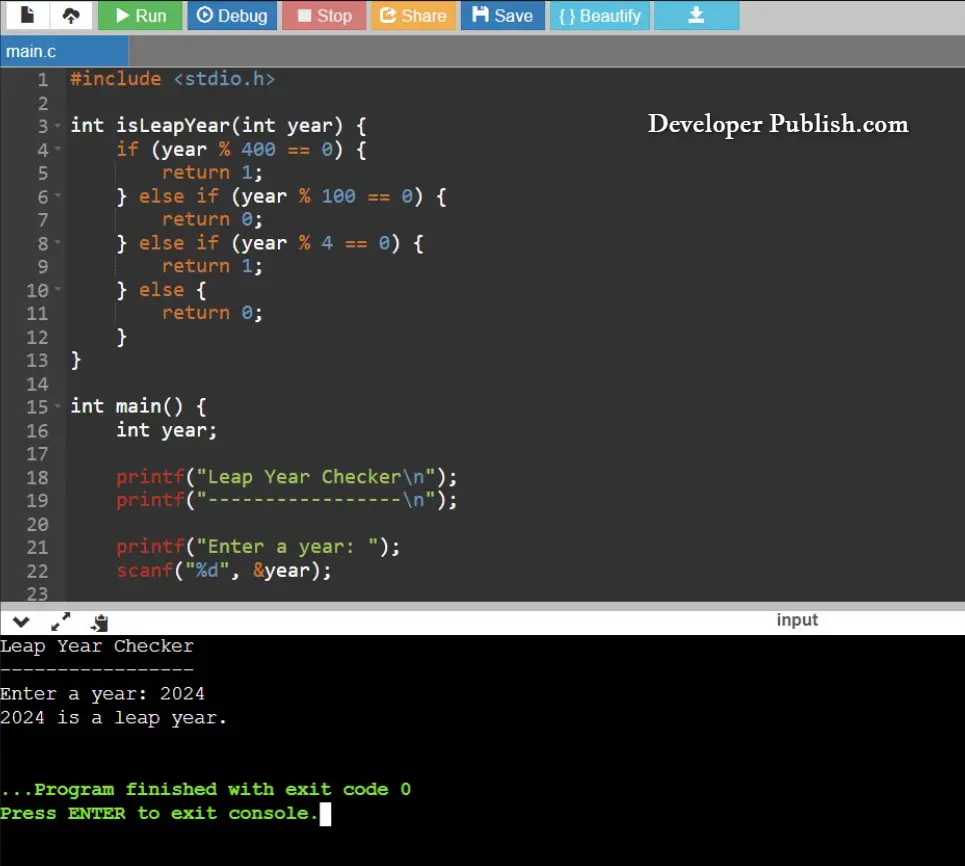