This C program converts time from the 12-hour format (HH:MM AM/PM) to the 24-hour format (HH:MM). It takes the input time in the 12-hour format from the user and converts it to the equivalent time in the 24-hour format.
Problem statement
Write a C program to convert time from the 12-hour format to the 24-hour format.
C Program to Convert Time from 12 Hour to 24 Hour Format
#include<stdio.h> void convertTo24HourFormat(int *hour, int *minute, char *period) { if (*period == 'P' || *period == 'p') { if (*hour != 12) { *hour = *hour + 12; } } else { if (*hour == 12) { *hour = 0; } } } int main() { int hour, minute; char period; printf("12-Hour Time Format to 24-Hour Time Format Conversion\n"); printf("----------------------------------------------------\n\n"); printf("Enter time in 12-hour format (HH:MM AM/PM): "); scanf("%d:%d %c", &hour, &minute, &period); convertTo24HourFormat(&hour, &minute, &period); printf("Equivalent time in 24-hour format: %02d:%02d\n", hour, minute); return 0; }
How it works?
The program takes the time in the 12-hour format as input from the user and converts it to the 24-hour format.
To perform the conversion, the program checks if the period is ‘P’ (indicating PM) or ‘p’. If it is PM and the hour is not already 12, it adds 12 to the hour to get the equivalent hour in the 24-hour format.
If the period is ‘A’ (indicating AM) or ‘a’, and the hour is 12, it sets the hour to 0 to get the equivalent hour in the 24-hour format.
The program then prints the equivalent time in the 24-hour format.
Input/Output
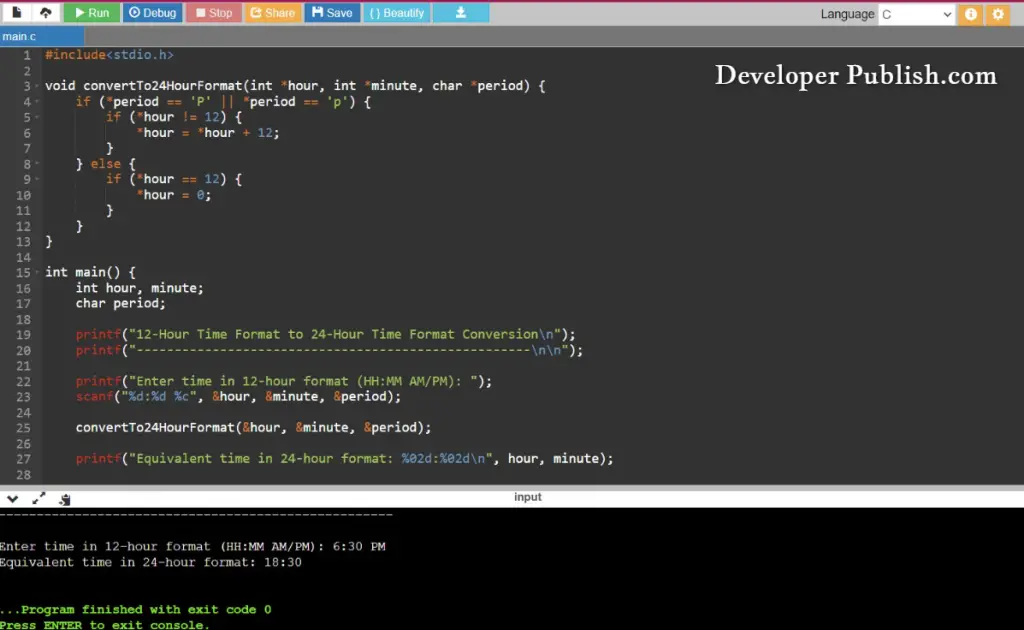