This C program uses recursion to reverse a given number. Recursion is a process in which a function calls itself as a subroutine. The reverseNumber
function is called recursively to reverse the digits of the input number.
Problem Statement
Given a positive integer, we need to reverse the digits of that number using recursion.
C Program to Reverse a Number using Recursion
#include <stdio.h> // Function to reverse a number using recursion int reverseNumber(int num) { static int reversedNum = 0; if (num == 0) { return 0; } reversedNum = (reversedNum * 10) + (num % 10); reverseNumber(num / 10); return reversedNum; } int main() { int number, reversedNumber; printf("Enter a positive integer: "); scanf("%d", &number); reversedNumber = reverseNumber(number); printf("The reversed number is: %d\n", reversedNumber); return 0; }
How it Works?
- The program defines a function
reverseNumber
that takes an integernum
as an argument and returns the reversed number. - The function uses a static variable
reversedNum
to keep track of the reversed number throughout the recursion. It is initially set to 0. - Inside the
reverseNumber
function, the base condition is checked. Ifnum
becomes 0, the function returns 0, ending the recursion. - If the base condition is not met, the function recursively calls itself with the updated value of
num
divided by 10. This helps in moving to the next digit of the input number. - During each recursive call, the function updates the
reversedNum
by multiplying it by 10 and adding the last digit ofnum
obtained using the modulus operator. - After the recursion ends, the final value of
reversedNum
is returned. - In the
main
function, the user is prompted to enter a positive integer. - The
scanf
function is used to read the input number and store it in thenumber
variable. - The
reverseNumber
function is called with thenumber
as an argument, and the returned value is stored in thereversedNumber
variable. - Finally, the reversed number is printed to the console.
Input/Output
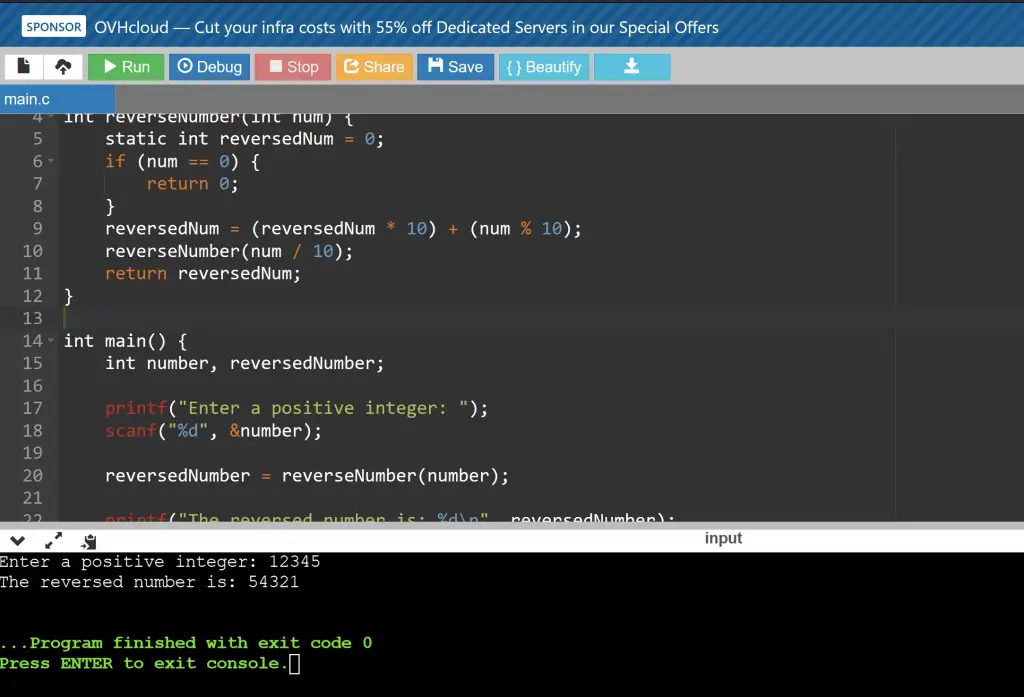