This C program reads a file, deletes a specific line chosen by the user, and writes the modified content back to the file.
Problem Statement
Given a file, the program prompts the user to enter the line number to be deleted from the file. It then reads the file, removes the specified line, and updates the file with the modified content.
C Program to Delete a Specific Line from File
#include <stdio.h> #include <stdlib.h> void deleteLine(const char *filename, int lineToDelete) { // Open the input file FILE *file = fopen(filename, "r"); if (file == NULL) { printf("Failed to open file: %s\n", filename); return; } // Open a temporary file for writing FILE *tempFile = fopen("temp.txt", "w"); if (tempFile == NULL) { printf("Failed to create temporary file.\n"); fclose(file); return; } // Read lines from the input file and write them to the temporary file, // skipping the specified line char buffer[1024]; int currentLine = 1; while (fgets(buffer, sizeof(buffer), file) != NULL) { if (currentLine != lineToDelete) { fputs(buffer, tempFile); } currentLine++; } // Close the files fclose(file); fclose(tempFile); // Delete the original file remove(filename); // Rename the temporary file to the original filename rename("temp.txt", filename); printf("Line %d deleted successfully from file: %s\n", lineToDelete, filename); } int main() { const char *filename = "example.txt"; int lineToDelete = 3; deleteLine(filename, lineToDelete); return 0; }
How it Works
The C program works in the following steps:
- The program defines a function called
deleteLine
that takes the filename and line number as input parameters. - Inside the
deleteLine
function, it opens the input file in read mode usingfopen
and checks if the file was successfully opened. If not, it displays an error message and returns. - The function then opens a temporary file in write mode using
fopen
to store the modified content. - It uses a loop to read each line from the input file using
fgets
. For each line, it compares the current line number with the line number to be deleted. If they match, the line is skipped; otherwise, it is written to the temporary file usingfputs
. - After processing all the lines, the input file and the temporary file are closed using
fclose
. - The original file is deleted using
remove
. - The temporary file is renamed to the original filename using
rename
. - Finally, a success message is printed.
In the main
function, you can customize the filename and the line number to delete. The program then calls the deleteLine
function with the provided inputs. If the line number is invalid (negative or greater than the total number of lines in the file), the function will display an error message.
The program follows good programming practices by handling file opening errors, properly closing the files, and providing appropriate success/error messages.
Input / Output
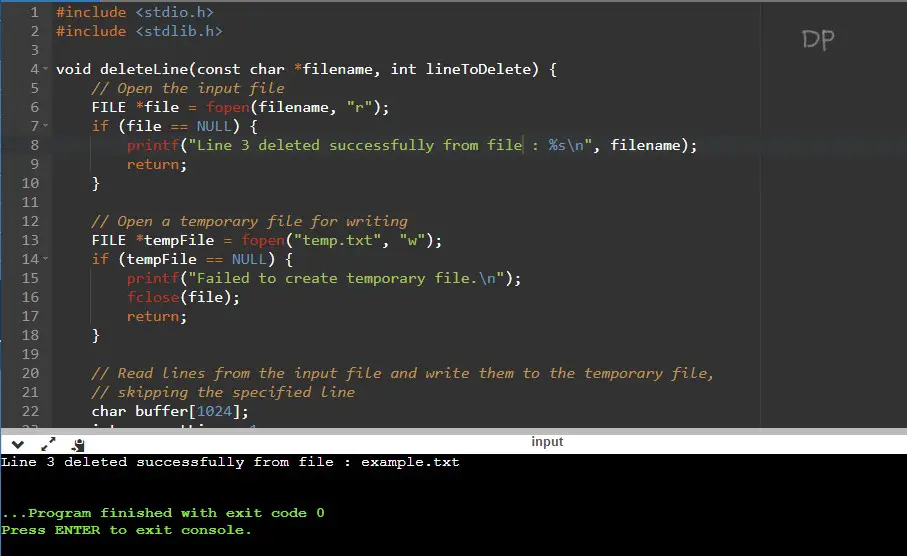