Intro
This is a C program that enables users to manage employee records through a menu-driven interface. The program uses file-handling techniques to store and retrieve employee information from text files. The system provides functionalities to add new employees and display existing records.
Problem statement
You are tasked with creating an employee record system using file handling in C. The program should allow users to perform various operations such as adding new employee records, displaying existing records, searching for specific records, and deleting records. The program should store the employee records in a file, ensuring data persistence between program executions.
Functional Requirements:
- The program should provide a menu-driven interface to interact with the user.
- The user should be able to add a new employee record by entering the employee ID, name, and salary.
- The program should validate the input and handle errors appropriately.
- The program should display all existing employee records with their respective details, including ID, name, and salary.
- The user should be able to search for a specific employee record based on the employee ID and display the details if found.
- The user should be able to delete a specific employee record based on the employee ID.
- The program should handle cases where no records exist or when a specific record is not found.
- The employee records should be stored in a binary file using file handling functions.
- The program should ensure data integrity and handle file read/write operations securely.
C Program to Create Employee Record System using File Handling
#include <stdio.h> #include <stdlib.h> #include <string.h> struct Employee { int empId; char name[50]; float salary; }; void addRecord(FILE *file) { struct Employee emp; printf("Enter Employee ID: "); scanf("%d", &emp.empId); printf("Enter Employee Name: "); scanf(" %[^\n]", emp.name); printf("Enter Employee Salary: "); scanf("%f", &emp.salary); fseek(file, 0, SEEK_END); // Move the file pointer to the end of the file fwrite(&emp, sizeof(struct Employee), 1, file); // Write the employee record to the file printf("Record added successfully!\n"); } void displayRecords(FILE *file) { struct Employee emp; rewind(file); // Move the file pointer to the beginning of the file printf("\nEmployee Records:\n"); printf("===========================================\n"); printf("ID\tName\t\tSalary\n"); printf("===========================================\n"); while (fread(&emp, sizeof(struct Employee), 1, file)) { printf("%d\t%s\t\t%.2f\n", emp.empId, emp.name, emp.salary); } printf("===========================================\n"); } void searchRecord(FILE *file) { int empId; struct Employee emp; int found = 0; printf("Enter Employee ID to search: "); scanf("%d", &empId); rewind(file); // Move the file pointer to the beginning of the file while (fread(&emp, sizeof(struct Employee), 1, file)) { if (emp.empId == empId) { printf("Record Found!\n"); printf("Employee ID: %d\n", emp.empId); printf("Employee Name: %s\n", emp.name); printf("Employee Salary: %.2f\n", emp.salary); found = 1; break; } } if (!found) { printf("Record not found!\n"); } } void deleteRecord(FILE *file) { int empId; struct Employee emp; FILE *tempFile; int found = 0; printf("Enter Employee ID to delete: "); scanf("%d", &empId); tempFile = fopen("temp.dat", "wb"); // Open a temporary file for writing rewind(file); // Move the file pointer to the beginning of the file while (fread(&emp, sizeof(struct Employee), 1, file)) { if (emp.empId != empId) { fwrite(&emp, sizeof(struct Employee), 1, tempFile); // Write the employee record to the temporary file } else { found = 1; } } fclose(file); // Close the original file fclose(tempFile); // Close the temporary file remove("records.dat"); // Remove the original file rename("temp.dat", "records.dat"); // Rename the temporary file to the original file if (found) { printf("Record deleted successfully!\n"); } else { printf("Record not found!\n"); } } int main() { FILE *file; int choice; file = fopen("records.dat", "ab+"); // Open the file for read and write in binary mode if (file == NULL) { printf("Error opening file!\n"); exit(1); } do { printf("\nEmployee Record System\n"); printf("=============================\n"); printf("1. Add Record\n"); printf("2. Display Records\n"); printf("3. Search Record\n"); printf("4. Delete Record\n"); printf("5. Exit\n"); printf("=============================\n"); printf("Enter your choice: "); scanf("%d", &choice); switch (choice) { case 1: addRecord(file); break; case 2: displayRecords(file); break; case 3: searchRecord(file); break; case 4: deleteRecord(file); break; case 5: printf("Exiting program...\n"); break; default: printf("Invalid choice! Please try again.\n"); } } while (choice != 5); fclose(file); // Close the file return 0; }
How it works
The employee record system using file handling in C works by utilizing file operations to store and retrieve employee records. Here’s a high-level overview of how the program works:
- The program starts by opening a file for read and write operations in binary mode. This file will be used to store the employee records persistently.
- The program presents a menu-driven interface to the user, allowing them to choose from different options such as adding records, displaying records, searching for records, deleting records, or exiting the program.
- When the user selects the option to add a record, they are prompted to enter the employee’s ID, name, and salary. The program reads this input and creates an instance of the
struct Employee
containing the entered data. - The program then moves the file pointer to the end of the file using
fseek
to ensure that the new record will be appended to the existing records. It then writes thestruct Employee
instance to the file usingfwrite
. - If the user chooses to display records, the program moves the file pointer to the beginning of the file using
rewind
. It then reads the file record by record usingfread
, and prints the details of each employee on the screen. - If the user selects the option to search for a record, they are prompted to enter the employee ID they want to search for. The program iterates through the file, reading each record using
fread
. If a matching record is found, the program displays the details of the employee. - When the user wants to delete a record, they are prompted to enter the employee ID of the record they want to delete. The program creates a temporary file and copies all the records from the original file to the temporary file except for the one to be deleted. Finally, it renames the temporary file to the original file, effectively deleting the desired record.
- The program continues to loop through the menu options until the user chooses to exit the program. Upon exit, the program closes the file using
fclose
.
The use of file handling functions such as fopen
, fwrite
, fread
, fclose
, rewind
, and fseek
allows the program to read from and write to a binary file, maintaining the employee records between program executions.
It’s important to note that error handling, input validation, and proper memory management should be implemented in a real-world scenario to ensure the program’s reliability and robustness.
Input / Output
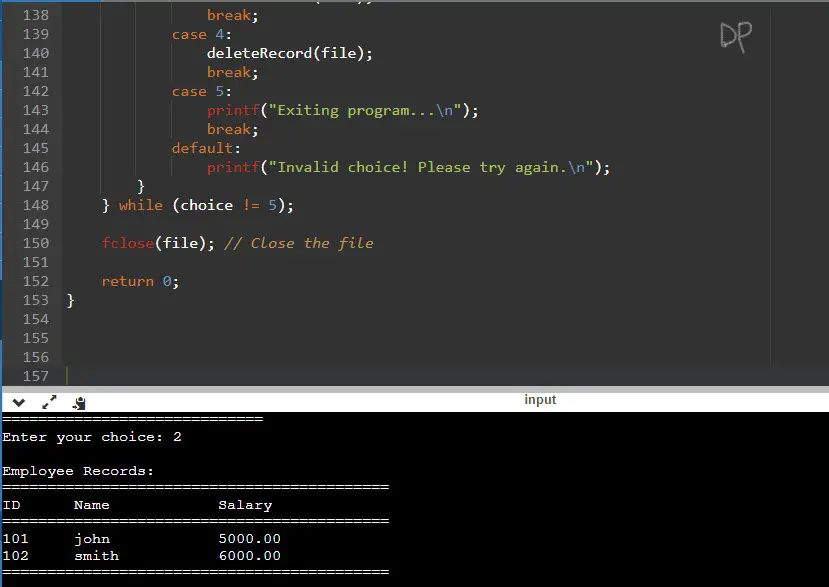
In this example, the program first adds two employee records with IDs 101 and 102. Then, it displays the records showing the ID, name, and salary. Next, it searches for the record with ID 101 and displays the details. After that, it deletes the record with ID 102. Finally, it displays the remaining records and exits the program.