This C program checks whether a given number is a palindrome or not.
What is a Palindrome?
A palindrome is a sequence of characters, numbers, or other elements that reads the same forward and backward. In the context of numbers, a palindrome number is a number that remains the same when its digits are reversed. you can easily determine whether a number is a palindrome or not. Palindrome numbers can be interesting to study and are often used in programming challenges and interview questions.
Problem Statement
Write a C program that checks whether a given number is a palindrome or not.
A palindrome number is a number that remains the same when its digits are reversed.
Ensure that your program handles both positive and negative numbers correctly.
C Program to Check Whether a Number is Palindrome or Not
#include <stdio.h> int isPalindrome(int number) { int reverse = 0; int original = number; while (number != 0) { int remainder = number % 10; reverse = reverse * 10 + remainder; number /= 10; } if (original == reverse) return 1; else return 0; } int main() { int number; printf("Enter a number: "); scanf("%d", &number); if (isPalindrome(number)) printf("%d is a palindrome.\n", number); else printf("%d is not a palindrome.\n", number); return 0; }
How it Works
- The program starts by prompting the user to enter a number.
- The input number is read from the user and stored in the variable
number
. - The program then calls the
isPalindrome
function with the input number as an argument. - Inside the
isPalindrome
function:- Two variables,
reverse
andoriginal
, are initialized to 0 andoriginal = number
, respectively. - A loop is used to reverse the digits of the number:
- The last digit of the number is extracted using the modulo operator (
remainder = number % 10
). - The
reverse
variable is updated by multiplying it by 10 and adding theremainder
. - The number is divided by 10 to remove the last digit (
number /= 10
). - These steps are repeated until the number becomes 0.
- The last digit of the number is extracted using the modulo operator (
- After the loop, the
reverse
andoriginal
variables are compared. - If they are equal, the function returns 1 to indicate that the number is a palindrome.
- If they are not equal, the function returns 0 to indicate that the number is not a palindrome.
- Two variables,
- The result of the
isPalindrome
function is stored in a variable calledisPal
. - The program then displays the appropriate message based on the value of
isPal
:- If
isPal
is 1, the program prints “<number> is a palindrome.” - If
isPal
is 0, the program prints “<number> is not a palindrome.”
- If
That’s how the program works! It checks whether a given number is a palindrome by reversing its digits and comparing the reversed number with the original number.
Input / Output
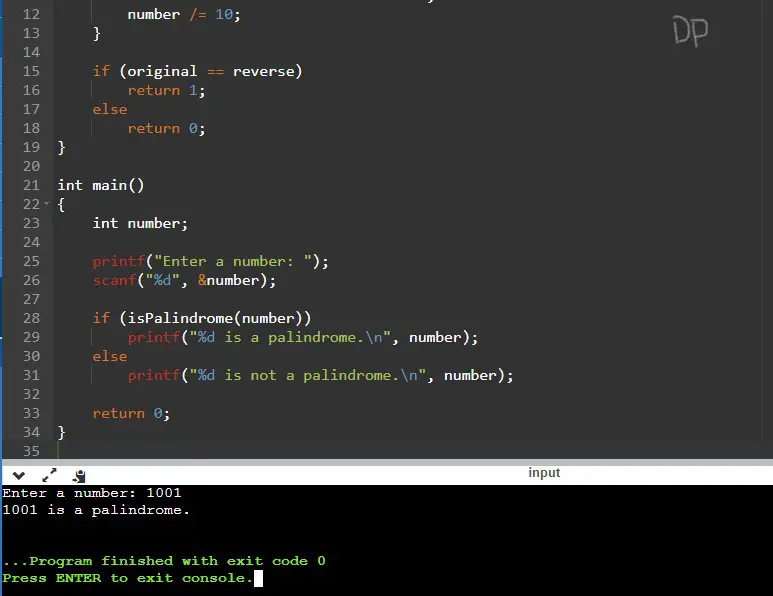