This C program checks whether a given integer has an alternate pattern, i.e., whether the binary representation of the integer consists of alternating 0s and 1s.
Problem Statement
Write a C program that checks whether a given integer has an alternate pattern of digits. The program should prompt the user to enter an integer and then determine if the digits in the number form an alternating pattern, where no two consecutive digits are the same. The program should output whether the given integer has an alternate pattern or not.
Specifications:
- The program should take an integer as input from the user.
- It should check if the digits in the number form an alternate pattern.
- An alternate pattern means that no two consecutive digits are the same.
- If the number has an alternate pattern, the program should print “The given integer has an alternate pattern.”
- If the number does not have an alternate pattern, the program should print “The given integer does not have an alternate pattern.”
C Program to Check whether the Given Integer has an Alternate Pattern
#include <stdio.h> int hasAlternatePattern(int num) { int prevDigit = num % 10; num /= 10; while (num > 0) { int currDigit = num % 10; if (currDigit == prevDigit) { return 0; // Not an alternate pattern } prevDigit = currDigit; num /= 10; } return 1; // Alternate pattern found } int main() { int num; printf("Enter an integer: "); scanf("%d", &num); if (hasAlternatePattern(num)) { printf("The given integer has an alternate pattern.\n"); } else { printf("The given integer does not have an alternate pattern.\n"); } return 0; }
How it Works
- The program prompts the user to enter an integer.
- The entered integer is passed to the
hasAlternatePattern
function. - Inside the
hasAlternatePattern
function, the rightmost digit of the number is extracted using the modulus operator (num % 10
), and this digit is stored as the previous digit. - The number is then divided by 10 (
num /= 10
) to remove the rightmost digit. - The process continues in a loop until the number becomes 0. In each iteration of the loop, the next rightmost digit is extracted and compared with the previous digit.
- If the current digit is the same as the previous digit, it means the pattern is not alternate, and the function returns 0.
- If no repeated adjacent digits are found during the loop, the function returns 1, indicating that the number has an alternate pattern.
- The return value from the
hasAlternatePattern
function is received in themain
function. - Based on the return value, the
main
function prints the appropriate message: “The given integer has an alternate pattern” or “The given integer does not have an alternate pattern.”
In summary, the program analyzes the digits of the entered integer by comparing each digit with its adjacent digit(s) to determine whether they form an alternate pattern. By iterating through the digits and checking for consecutive repeats, the program can identify if the integer exhibits the desired pattern.
Input / Output
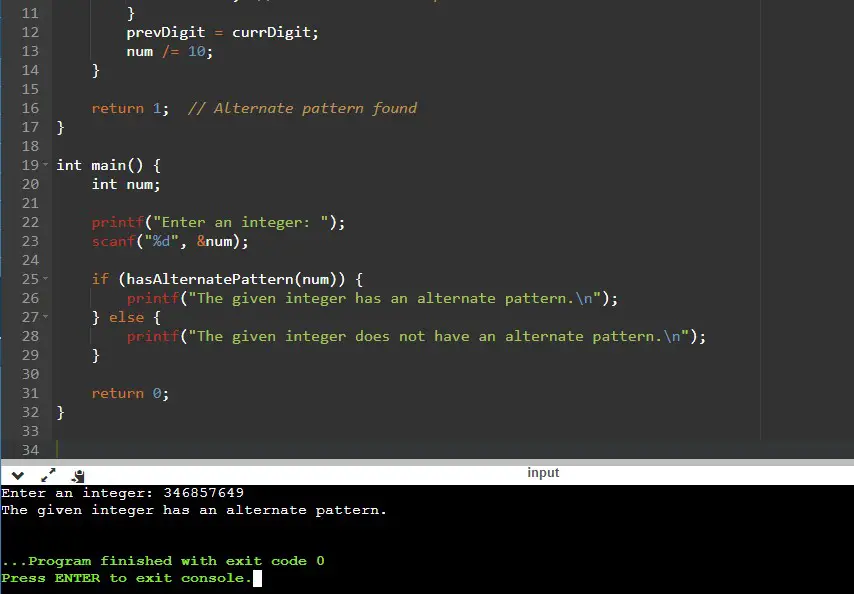
Explanation: The digits in the number 346857649 are 3,4,6,8,5,7,6,4 and 9, which form an alternate pattern.