This C program finds the frequency of a substring in a given string.
Problem statement
You are required to write a C program that calculates the frequency of a given substring within a given string.
Your program should prompt the user to enter a string and a substring. The program will then search for the substring within the string and determine how many times the substring appears.
C Program to Find the Frequency of a Substring in a String
#include <stdio.h> #include <string.h> int countSubstring(const char *string, const char *substring) { int count = 0; int substringLength = strlen(substring); int stringLength = strlen(string); if (substringLength > stringLength) { return 0; } for (int i = 0; i <= stringLength - substringLength; i++) { int j; for (j = 0; j < substringLength; j++) { if (string[i + j] != substring[j]) { break; } } if (j == substringLength) { count++; } } return count; } int main() { char string[100]; char substring[50]; printf("Enter a string: "); fgets(string, sizeof(string), stdin); printf("Enter a substring: "); fgets(substring, sizeof(substring), stdin); // Removing trailing newline characters string[strcspn(string, "\n")] = '\0'; substring[strcspn(substring, "\n")] = '\0'; int frequency = countSubstring(string, substring); printf("Frequency of substring in the string: %d\n", frequency); return 0; }
How it works
- The program starts by defining the necessary header files, including
stdio.h
for input/output operations andstring.h
for string manipulation functions. - The
countSubstring
function is declared. It takes two arguments:string
(the main string to search in) andsubstring
(the string to search for). It returns an integer value representing the frequency of the substring in the string. - Inside the
countSubstring
function, several variables are declared:count
to keep track of the frequency,substringLength
to store the length of the substring, andstringLength
to store the length of the main string. - If the length of the substring is greater than the length of the string, it means the substring cannot be found, so the function immediately returns 0.
- The function uses two nested loops to compare each character of the substring with the corresponding characters in the string. The outer loop iterates through each character position in the string.
- Inside the outer loop, the inner loop iterates through each character of the substring and checks for a match with the corresponding character in the string. If a mismatch is found, the inner loop breaks, and the outer loop continues to the next position in the string.
- If the inner loop completes without any mismatches, it means the substring is found at that position in the string. The
count
variable is incremented. - After checking all possible positions in the string, the final
count
is returned. - In the
main
function, variablesstring
andsubstring
are declared to store the user input. - The user is prompted to enter a string using
printf
. Thefgets
function is then used to read the input from the user and store it in thestring
variable. - The user is prompted to enter a substring in a similar manner, and the input is stored in the
substring
variable. - Next,
strcspn
is used to remove any trailing newline characters from thestring
andsubstring
. - The
countSubstring
function is called with thestring
andsubstring
as arguments, and the result is stored in thefrequency
variable. - Finally, the program prints the frequency of the substring in the string using
printf
.
That’s how the program works! It takes user input, searches for the substring in the string, and calculates the frequency of its occurrence
Input / Output
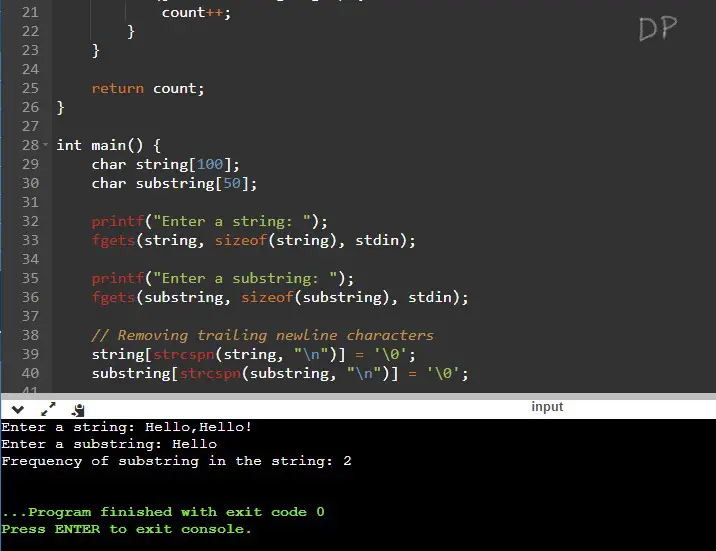