Tic Tac Toe is a classic paper-and-pencil game played on a 3×3 grid. The objective of the game is to get three of your own symbols (traditionally X or O) in a row, either horizontally, vertically, or diagonally, before your opponent does.
Problem statement
You are tasked with implementing a Tic Tac Toe game in C Programming. The game should be played between two players, X and O, on a 3×3 grid. The players take turns marking empty cells on the grid until one of them wins or the game ends in a tie.
Here are the requirements for your program:
- Display an empty 3×3 grid at the beginning of the game.
- Alternate between the two players (X and O) and allow them to enter their moves.
- Validate the player’s input to ensure it represents a valid empty cell on the grid.
- Update the grid with the player’s mark (X or O) in the chosen cell.
- After each move, check if the current player has won the game. A player wins if they have three of their marks in a row, column, or diagonal.
- If a player wins, display a message announcing the winner and end the game.
- If the game ends in a tie (all cells are filled and no player has won), display a message announcing the tie.
- Continue the game until a player wins or it ends in a tie.
- Display the final grid at the end of the game
C Program demonstrating Tic Tac Toe Game
#include <stdio.h> // Function to display the Tic Tac Toe board void displayBoard(char board[3][3]) { printf("-------------\n"); for (int i = 0; i < 3; i++) { printf("| %c | %c | %c |\n", board[i][0], board[i][1], board[i][2]); printf("-------------\n"); } } // Function to check if a player has won int checkWin(char board[3][3], char player) { // Check rows for (int i = 0; i < 3; i++) { if (board[i][0] == player && board[i][1] == player && board[i][2] == player) return 1; } // Check columns for (int i = 0; i < 3; i++) { if (board[0][i] == player && board[1][i] == player && board[2][i] == player) return 1; } // Check diagonals if (board[0][0] == player && board[1][1] == player && board[2][2] == player) return 1; if (board[0][2] == player && board[1][1] == player && board[2][0] == player) return 1; return 0; } int main() { char board[3][3] = {{' ', ' ', ' '}, {' ', ' ', ' '}, {' ', ' ', ' '}}; // Empty board int row, col; char currentPlayer = 'X'; printf("Tic Tac Toe Game\n"); // Main game loop while (1) { displayBoard(board); // Get the player's move printf("Player %c, enter your move (row [1-3] column [1-3]): ", currentPlayer); scanf("%d %d", &row, &col); // Convert the input to array indices row--; col--; // Check if the move is valid if (row < 0 || row >= 3 || col < 0 || col >= 3 || board[row][col] != ' ') { printf("Invalid move! Try again.\n"); continue; } // Make the move board[row][col] = currentPlayer; // Check if the current player has won if (checkWin(board, currentPlayer)) { printf("Player %c wins!\n", currentPlayer); break; } // Check if it's a tie int isTie = 1; for (int i = 0; i < 3; i++) { for (int j = 0; j < 3; j++) { if (board[i][j] == ' ') { isTie = 0; break; } } if (!isTie) break; } if (isTie) { printf("It's a tie!\n"); break; } // Switch to the other player currentPlayer = (currentPlayer == 'X') ? 'O' : 'X'; } displayBoard(board); return 0; }
How it works
- The program starts by initializing an empty 3×3 board using a 2-dimensional array called
board
. - The
displayBoard
function is defined to print the current state of the board, including the player marks. - The
checkWin
function is defined to check if a player has won the game. It checks for winning conditions in rows, columns, and diagonals. - In the
main
function, the main game loop begins. It continues indefinitely until a player wins or the game ends in a tie. - Inside the loop, the
displayBoard
function is called to show the current state of the board. - The current player is prompted to enter their move by specifying the row and column numbers.
- The input is validated to ensure it represents a valid empty cell on the board. If the move is invalid, an error message is displayed, and the loop continues to the next iteration.
- If the move is valid, the board is updated with the current player’s mark (X or O) in the chosen cell.
- After each move, the
checkWin
function is called to check if the current player has won the game. If a player wins, a congratulatory message is displayed, and the game loop is terminated. - If the game has not ended in a win, the program checks for a tie condition. If all cells on the board are filled and no player has won, a tie message is displayed, and the game loop is terminated.
- If the game is still ongoing, the turn is switched to the other player by assigning the value of ‘O’ to currentPlayer if it was ‘X’, or vice versa.
- Finally, after the game loop ends, the
displayBoard
function is called one last time to show the final state of the board.
That’s how the program works! It keeps track of the game state, validates moves, checks for wins or ties, and provides an interactive interface for players to play Tic Tac Toe
Input / Output
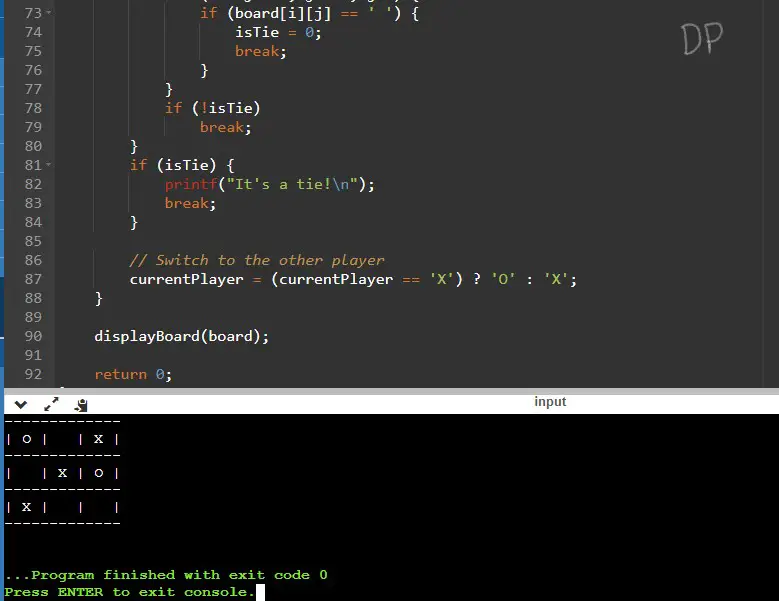