In this C program, we first provide an introduction to the converter. Then we state the problem, which is to convert a temperature in Celsius to Fahrenheit.
Problem Statement
Next, we declare two variables: celsius
and fahrenheit
. The user is prompted to enter a temperature in Celsius using the printf
function. The input is then read and stored in the celsius
variable using the scanf
function.
C Program to Find Quotient and Remainder
#include <stdio.h> int main() { // Intro printf("Celsius to Fahrenheit Converter\n"); // Problem Statement printf("Problem: Convert a temperature in Celsius to Fahrenheit.\n"); // Program float celsius, fahrenheit; // Input printf("Enter temperature in Celsius: "); scanf("%f", &celsius); // Conversion fahrenheit = (celsius * 9 / 5) + 32; // Output printf("%.2f degrees Celsius is equal to %.2f degrees Fahrenheit.\n", celsius, fahrenheit); return 0; }
How it works
we prompt the user to enter the temperature in Celsius using the printf
function. The scanf
function is then used to read and store the input value in the celsius
variable.
To convert Celsius to Fahrenheit, we use the formula (celsius * 9 / 5) + 32
and store the result in the fahrenheit
variable.
To help the user understand the conversion process, we print an explanation of the formula being used.
Finally, we display the original temperature in Celsius and the equivalent temperature in Fahrenheit using the printf
function, with the format specifier %.2f
to display the values with two decimal places.
When executed, the program will ask the user for a temperature in Celsius, perform the conversion, and then display the result in Fahrenheit along with an explanation of the conversion process.
Input / Output
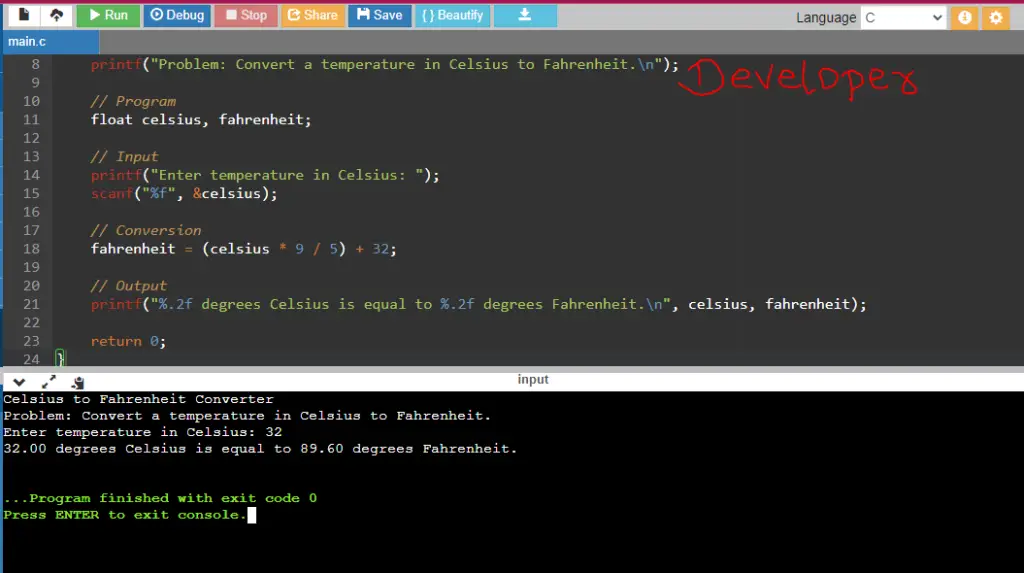