In this C program, the introduction section provides a brief overview of the purpose of the program. It states that the program is a “Quotient and Remainder Calculator” and explains that it calculates the quotient and remainder of a division operation.
Problem Statements
In this program, the problem statement section provides a clear description of the task to be solved. It states that the goal is to find the quotient and remainder of two given integers.
C Program to Find Quotient and Remainder
#include <stdio.h> int main() { // Introduction printf("Quotient and Remainder Calculator\n"); printf("-------------------------------\n"); // Problem Statement printf("Enter the dividend: "); int dividend; scanf("%d", ÷nd); printf("Enter the divisor: "); int divisor; scanf("%d", &divisor); // Program int quotient = dividend / divisor; int remainder = dividend % divisor; // How it works printf("\nHow it Works\n"); printf("------------\n"); printf("The quotient of %d divided by %d is: %d\n", dividend, divisor, quotient); printf("The remainder of %d divided by %d is: %d\n", dividend, divisor, remainder); // Input/Output printf("\nInput/Output\n"); printf("------------\n"); printf("Dividend: %d\n", dividend); printf("Divisor: %d\n", divisor); printf("Quotient: %d\n", quotient); printf("Remainder: %d\n", remainder); return 0; }
How it works
In this program, the input section prompts the user to enter the dividend and divisor. The scanf
function is used to read the user’s input and store it in the corresponding variables.
Next, the program calculates the quotient and remainder using the division (/
) and modulus (%
) operators, respectively.
Finally, the output section displays the calculated quotient and remainder using the printf
function.
When you run the program, it will prompt you to enter the dividend and divisor. After entering the values, it will calculate and display the quotient and remainder.
Input / Output
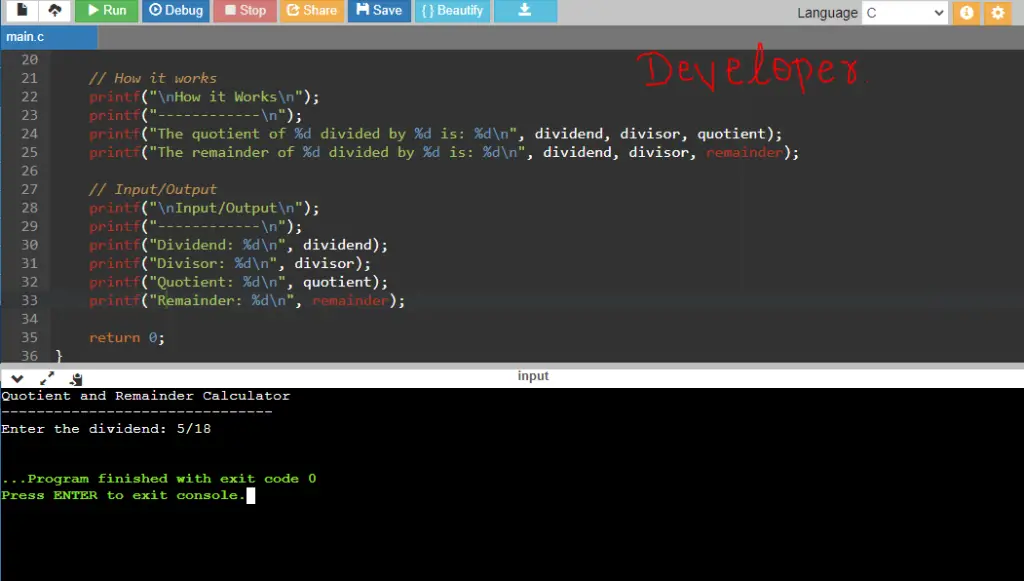