In C programming, a union is a user-defined data type that allows storing different data types in the same memory location. The size of a union is determined by the size of its largest member. In this program, we will calculate the size of a union.
Problem statement
Write a C program to find the size of a union.
C Program to Find the Size of a Union
#include <stdio.h> union Data { int num; float fraction; char character; }; int main() { union Data data; int size; size = sizeof(data); printf("Size of the union: %d bytes\n", size); return 0; }
how it works
- We start by including the necessary header file
stdio.h
to use theprintf
function. - We define a union named
Data
with three members:num
of typeint
,fraction
of typefloat
, andcharacter
of typechar
. - In the
main
function, we declare a variabledata
of typeunion Data
and an integer variablesize
. - Using the
sizeof
operator, we calculate the size of thedata
union and assign it to thesize
variable. - Finally, we print the size of the union using the
printf
function.
Input/Output
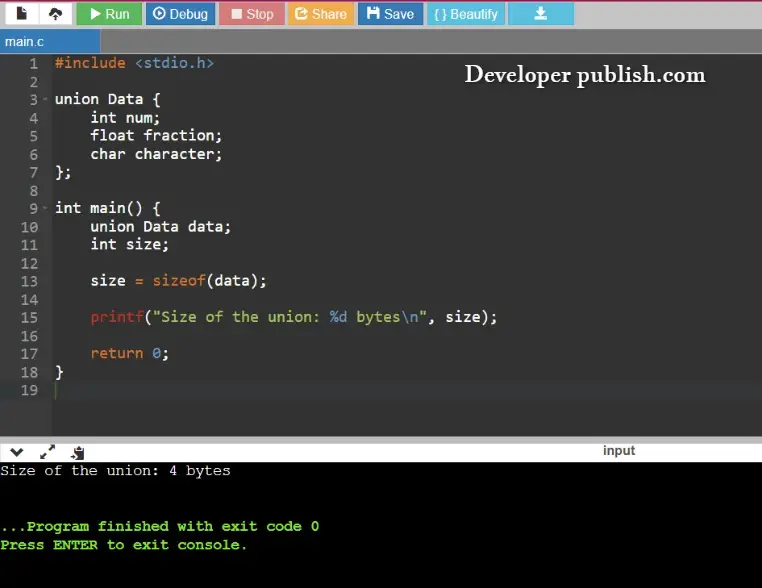