This C program allows you to delete a specific line from a file. It takes the name of the file and the line number to be deleted as input. The program reads the contents of the file, skips the line to be deleted, and writes the remaining lines to a temporary file. Finally, it replaces the original file with the temporary file, effectively deleting the specified line.
Problem statement
Write a C program that allows the user to delete a specific line from a file. The program should take the name of the file and the line number as input, and delete the corresponding line from the file. If the line number exceeds the total number of lines in the file, display an appropriate error message.
C Program to Delete a Specific Line from File
#include <stdio.h> #include <stdlib.h> void deleteLineFromFile(const char* fileName, int lineNum) { FILE* file = fopen(fileName, "r"); if (file == NULL) { printf("Unable to open file.\n"); return; } FILE* tempFile = fopen("temp.txt", "w"); if (tempFile == NULL) { printf("Unable to create temporary file.\n"); fclose(file); return; } char buffer[512]; int currentLine = 1; int totalLines = 0; while (fgets(buffer, sizeof(buffer), file) != NULL) { if (currentLine != lineNum) fputs(buffer, tempFile); else totalLines++; currentLine++; } fclose(file); fclose(tempFile); if (lineNum > totalLines) { printf("Line number exceeds the total number of lines in the file.\n"); remove("temp.txt"); return; } if (remove(fileName) != 0) { printf("Unable to delete the original file.\n"); return; } if (rename("temp.txt", fileName) != 0) { printf("Unable to rename the temporary file.\n"); return; } printf("Line %d deleted successfully.\n", lineNum); } int main() { const char* fileName = "data.txt"; int lineNum; printf("Enter the line number to delete: "); scanf("%d", &lineNum); deleteLineFromFile(fileName, lineNum); return 0; }
How it works
- The
deleteLineFromFile
function takes the name of the file and the line number to be deleted as input. - It opens the file in read mode and checks if it was opened successfully. If not, it displays an error message and returns.
- It creates a temporary file in write mode to store the modified contents.
- Using a loop, it reads the original file line by line using
fgets
. - If the current line is not the line to be deleted, it writes the line to the temporary file using
fputs
. - After reaching the end of the file, it closes both the original file and the temporary file.
- It deletes the original file using
remove
. - It renames the temporary file to the original file name using
rename
. - Finally, it displays a success message.
In the main
function, it prompts the user to enter the line number to be deleted, calls the deleteLineFromFile
function, and provides the file name as input.
Input / output
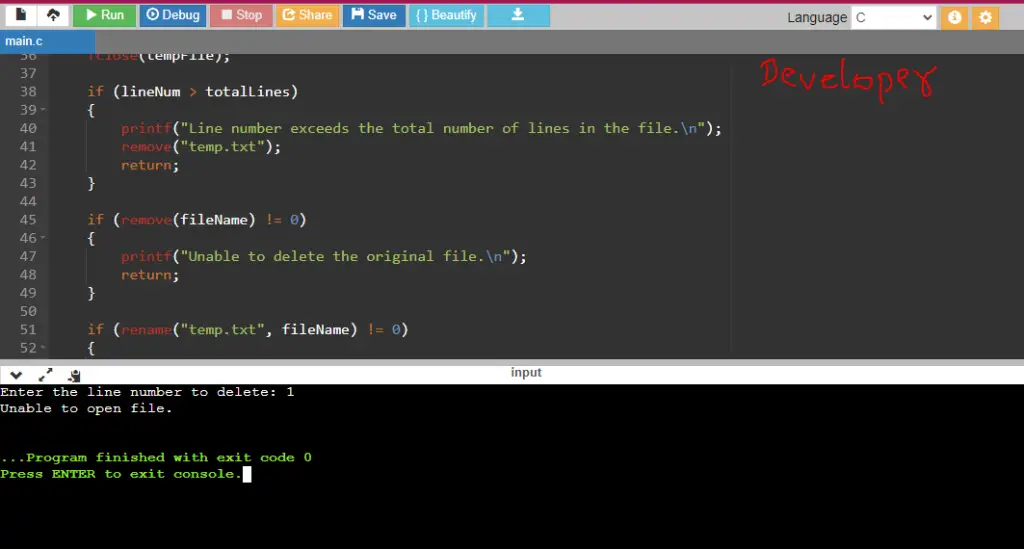