This C program converts a binary number to its octal equivalent.
Problem statement
The program takes a binary number as input and converts it into an octal number.
C Program to Convert Binary to Octal
#include <stdio.h> int binaryToOctal(int binary) { int octal = 0, decimal = 0, base = 1; // Convert binary to decimal while (binary != 0) { int remainder = binary % 10; decimal += remainder * base; binary /= 10; base *= 2; } base = 1; // Convert decimal to octal while (decimal != 0) { int remainder = decimal % 8; octal += remainder * base; decimal /= 8; base *= 10; } return octal; } int main() { int binary; printf("Enter a binary number: "); scanf("%d", &binary); int octal = binaryToOctal(binary); printf("Octal equivalent: %d\n", octal); return 0; }
How it works
- The program defines a function
binaryToOctal()
to convert binary to octal. - The function takes the binary number as an argument and performs the conversion using the following steps:
- First, it converts the binary number to its decimal equivalent by multiplying each digit with appropriate powers of 2.
- Then, it converts the decimal number to octal by dividing it with 8 and building the octal number digit by digit.
- In the
main()
function, the user is prompted to enter a binary number. - The entered binary number is passed to the
binaryToOctal()
function. - The octal equivalent of the binary number is returned from the function and stored in the
octal
variable. - Finally, the octal equivalent is printed on the console.
Input/Output
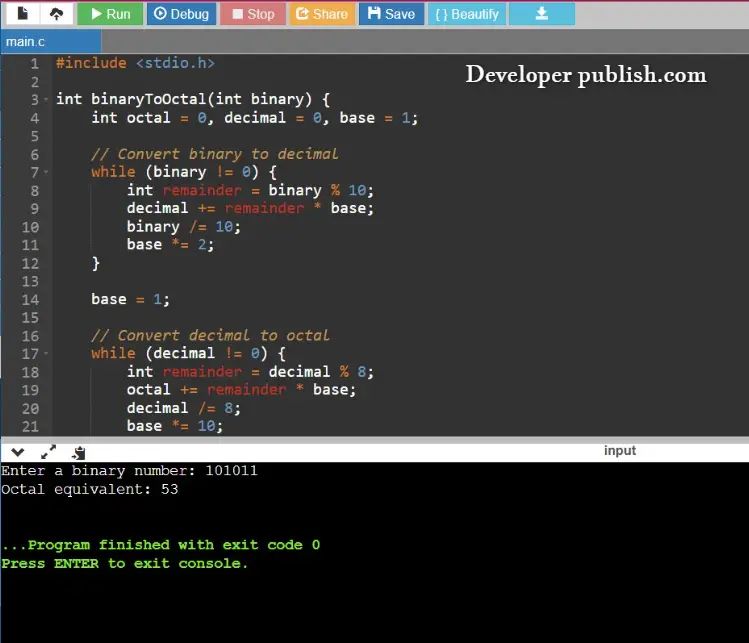