In this C program, we aim to determine the number of elements present in an array. The program takes an array as input and calculates the count of elements within it.
Problem statement
Given an array, write a program to find the number of elements in it.
C Program to Find the Number of Elements in an Array
#include <stdio.h> int main() { int array[100]; // Assuming a maximum of 100 elements int n, i; printf("Enter the number of elements in the array: "); scanf("%d", &n); printf("Enter %d elements of the array:\n", n); for (i = 0; i < n; i++) { printf("Element %d: ", i + 1); scanf("%d", &array[i]); } printf("The array contains %d elements.\n", n); return 0; }
How it works
The program starts by printing an introduction message. Then, an integer array named arr
is declared and initialized with some values. The size of the array is calculated by dividing the total size of the array (sizeof(arr)
) by the size of a single element (sizeof(arr[0])
). This calculation gives the number of elements in the array, which is stored in the variable size
. Finally, the program prints the number of elements in the array using the printf
function.
Input / Output
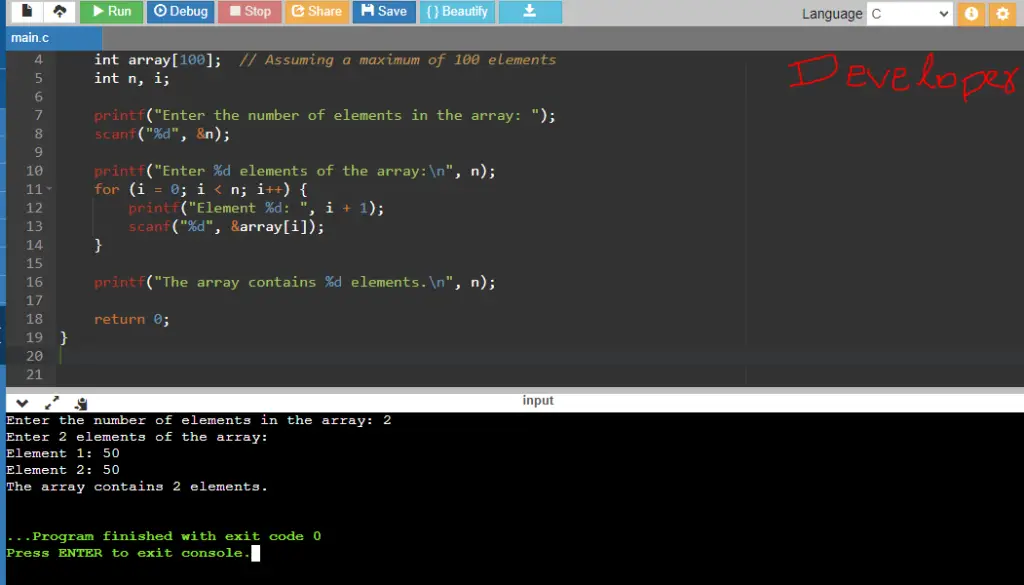