This C program calculates the area of a triangle using the formula: area = 0.5 * base * height.
Problem statement
Write a C program to find the area of a triangle using the given base length and height.
C Program to Find the Area of a Triangle
#include <stdio.h> int main() { printf("Program to calculate the area of a triangle\n"); float base, height; printf("Enter the base length of the triangle: "); scanf("%f", &base); printf("Enter the height of the triangle: "); scanf("%f", &height); float area = 0.5 * base * height; printf("The area of the triangle is: %f\n", area); return 0; }
How it works
- The program starts with an introduction message.
- It declares two variables:
base
andheight
to store the base length and height of the triangle, respectively. - It prompts the user to enter the base length of the triangle.
- The user enters the base length, which is read using the
scanf
function and stored in the variablebase
. - It prompts the user to enter the height of the triangle.
- The user enters the height, which is read using the
scanf
function and stored in the variableheight
. - The program calculates the area of the triangle using the formula
area = 0.5 * base * height
and stores the result in the variablearea
. - Finally, it displays the area of the triangle using the
printf
function.
Input / output
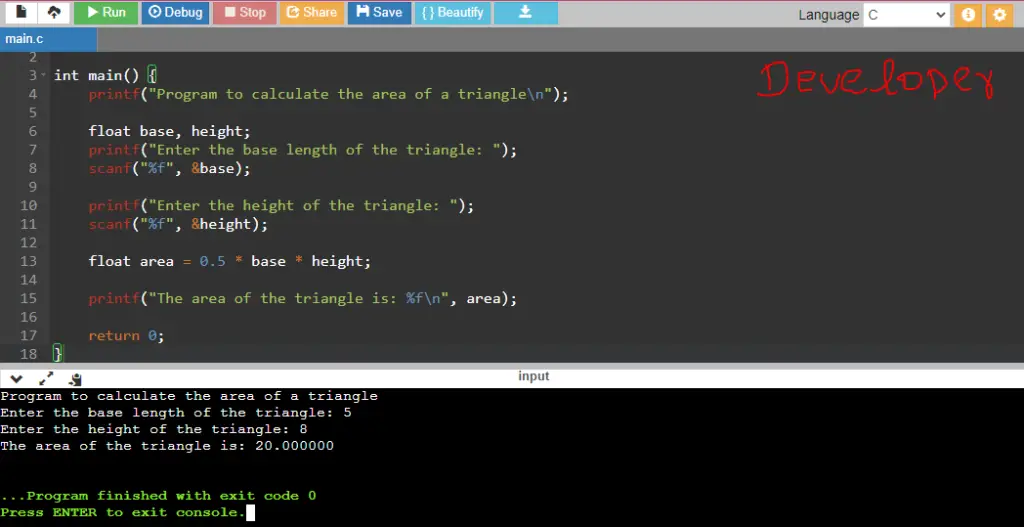