This C# program retrieves daylight saving time information for a specified time zone using the TimeZoneInfo
class. Daylight saving time (DST) is a practice in some regions where the local time is adjusted forward or backward by one hour during certain parts of the year to make better use of daylight.
Problem statement
Your task is to develop a C# program that retrieves and displays Daylight Saving Time (DST) information for the local time zone. The program should be able to determine whether the local time zone supports DST, and if so, provide details such as the standard time name, daylight saving time name, as well as the start and end dates of DST.
C# Program to Get the DayLight Saving Information
using System; class Program { static void Main() { // Get the local time zone TimeZoneInfo localTimeZone = TimeZoneInfo.Local; // Check if the time zone supports DST if (localTimeZone.SupportsDaylightSavingTime) { Console.WriteLine("Daylight Saving Time Information:"); Console.WriteLine("Standard Time Name: " + localTimeZone.StandardName); Console.WriteLine("Daylight Saving Time Name: " + localTimeZone.DaylightName); // Get the date when DST starts and ends TimeZoneInfo.AdjustmentRule[] adjustmentRules = localTimeZone.GetAdjustmentRules(); foreach (TimeZoneInfo.AdjustmentRule rule in adjustmentRules) { Console.WriteLine("DST Starts On: " + rule.DateStart.ToString()); Console.WriteLine("DST Ends On: " + rule.DateEnd.ToString()); } } else { Console.WriteLine("This time zone does not support Daylight Saving Time."); } } }
How it works
Let’s discuss how the C# program for retrieving Daylight Saving Time (DST) information works based on the problem statement:
- Initialization: The program starts by initializing the necessary components, including the use of the
TimeZoneInfo
class to work with time zone information. - Local Time Zone: The program uses
TimeZoneInfo.Local
to obtain information about the local time zone. This step ensures that the DST information retrieved is specific to the user’s system. - DST Support Check: It checks whether the local time zone supports Daylight Saving Time by using the
SupportsDaylightSavingTime
property of theTimeZoneInfo
object. - Displaying Information:
- If DST is supported:
- The program retrieves and displays the standard time name and daylight saving time name using the
StandardName
andDaylightName
properties. - It also accesses the
GetAdjustmentRules()
method to obtain a collection ofAdjustmentRule
objects that define the DST rules for the time zone. - For each
AdjustmentRule
, the program extracts the start and end dates of DST using theDateStart
andDateEnd
properties and displays them.
- The program retrieves and displays the standard time name and daylight saving time name using the
- If DST is not supported:
- The program outputs a message indicating that the local time zone does not support Daylight Saving Time.
- If DST is supported:
- Output: The program prints the retrieved information to the console, providing the user with details about DST if it’s supported in their time zone.
- Completion: The program completes its execution, and the DST information is displayed to the user.
By following these steps, the C# program effectively retrieves and displays Daylight Saving Time information for the local time zone, offering users insight into the DST rules and schedule applicable to their region.
Input/Output
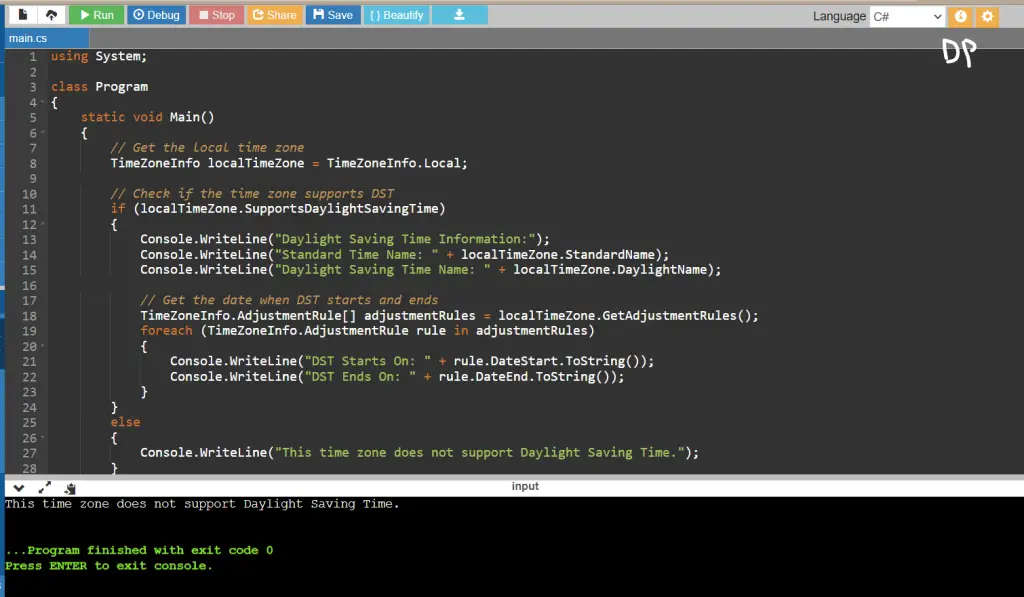