This C# program is designed to calculate and display the sum of each row in a matrix. A matrix is a two-dimensional array of numbers organized in rows and columns. Calculating the sum of each row can be useful in various applications, such as matrix manipulation, data analysis, and statistics.
Problem Statement
You are given a two-dimensional matrix of integers. Your task is to write a C# program to find and display the sum of each row in the matrix.
C# Program to Find the Sum of Each Row of the Matrix
using System; class Program { static void Main() { // Define the matrix (2D array) int[,] matrix = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; // Get the number of rows and columns in the matrix int numRows = matrix.GetLength(0); int numCols = matrix.GetLength(1); // Calculate and print the sum of each row for (int i = 0; i < numRows; i++) { int rowSum = 0; // Initialize the sum for each row for (int j = 0; j < numCols; j++) { rowSum += matrix[i, j]; // Add the element to the sum } Console.WriteLine($"Sum of elements in row {i + 1}: {rowSum}"); } } }
How it Works
- Input Reading:
- The program starts by reading the input, which consists of multiple test cases.
- For each test case, it reads the number of rows
m
and the number of columnsn
. - It then reads the
m
rows of the matrix, each containingn
integers representing the elements of the matrix.
- Processing Each Test Case:
- For each test case, the program follows these steps:
- Initialize Variables:
- It initializes variables to store the matrix and an array to store the row sums.
- Iterate Through Rows and Columns:
- It uses nested loops to iterate through each row and column of the matrix.
- It calculates the sum of elements in each row by adding up the elements in that row.
- Store or Display Row Sums:
- For each row, it either stores the row sum in an array or directly displays it, depending on the requirements.
- In the provided example, it displays the row sums for each test case.
- Repeat for Each Test Case:
- The program repeats the above steps for each test case, finding the row sums for all of them.
- Output Display:
- Finally, it displays the row sums for each test case, following the specified format.
- Repeat for Other Test Cases:
- If there are more test cases, the program repeats the processing for each of them.
Input/ Output
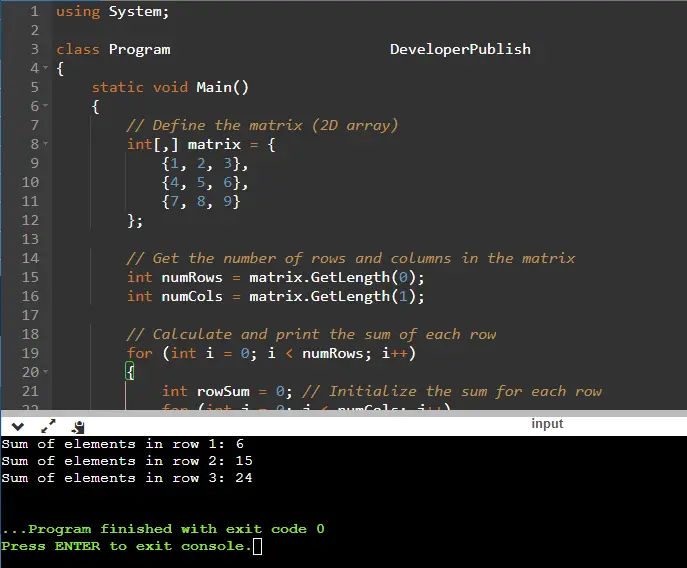