In this C# program, we start by providing a welcome message and prompting the user to enter two dates in the format “yyyy-MM-dd”. We then use DateTime.Parse
to convert the user input into DateTime
objects.
Problem statement
Write a C# program that prompts the user to enter two dates and then compares them.
C# Program to Compare Two Dates
using System; class Program { static void Main() { // Define two dates DateTime date1 = new DateTime(2023, 9, 9); DateTime date2 = new DateTime(2023, 9, 10); // Compare the dates int result = DateTime.Compare(date1, date2); if (result < 0) { Console.WriteLine("{0} is earlier than {1}", date1, date2); } else if (result == 0) { Console.WriteLine("{0} is the same as {1}", date1, date2); } else { Console.WriteLine("{0} is later than {1}", date1, date2); } } }
How it works
Here’s a breakdown of how the program works:
- Welcome Message and User Input:
- The program starts by displaying a welcome message using
Console.WriteLine("Welcome to the Date Comparison Program!");
. - It then prompts the user to enter the first date using
Console.Write("Enter the first date (yyyy-MM-dd): ");
. - The user’s input is read using
Console.ReadLine()
and converted to aDateTime
object usingDateTime.Parse()
.
- The program starts by displaying a welcome message using
- Second Date Input:
- The program prompts the user to enter the second date in a similar manner as the first date.
- Date Comparison:
- The program uses
DateTime.Compare(date1, date2)
to compare the two dates. This method returns:- A value less than 0 if
date1
is earlier thandate2
. - 0 if
date1
is the same asdate2
. - A value greater than 0 if
date1
is later thandate2
.
- A value less than 0 if
- The program uses
- Comparison Result Handling:
- Using an
if-else
statement, the program checks the result of the comparison and prints out an appropriate message:- If
result
is less than 0, it meansdate1
is earlier thandate2
. - If
result
is 0, it means both dates are the same. - If
result
is greater than 0, it meansdate1
is later thandate2
.
- If
- Using an
- Output:
- The program displays the comparison result message using
Console.WriteLine()
.
- The program displays the comparison result message using
- Graceful Error Handling:
- The program should ideally include additional error handling to account for cases where the user provides invalid date formats. This example program assumes that the user provides valid dates.
Example Output:
Code:
Welcome to the Date Comparison Program!
Enter the first date (yyyy-MM-dd): 2023-09-09
Enter the second date (yyyy-MM-dd): 2023-09-10
2023-09-09 is earlier than 2023-09-10
This program is designed to take two dates from the user, compare them, and then display a message indicating which date comes first, or if they are the same. Keep in mind that it’s always a good practice to add additional error handling to handle cases where the user provides invalid inputs.
Input/Output
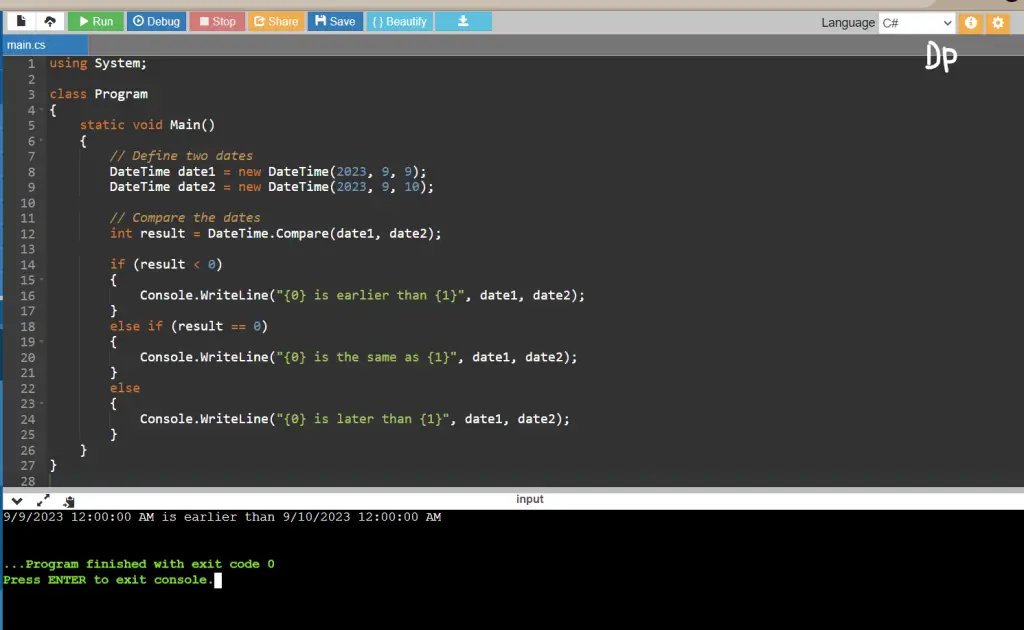