This C# program is designed to determine whether a given number is an Armstrong number or no
Problem Statement
An Armstrong number (also known as a narcissistic number or a pluperfect digital invariant) is a number that is equal to the sum of its own digits, each raised to the power of the number of digits in the number. Your task is to write a program that checks whether a given positive integer is an Armstrong number or not.
C# Program to Check Armstrong Number
using System; class ArmstrongNumberChecker { // Function to calculate the power of a number static int Power(int baseNumber, int exponent) { int result = 1; for (int i = 0; i < exponent; i++) { result *= baseNumber; } return result; } // Function to check if a number is an Armstrong number static bool IsArmstrongNumber(int number) { int originalNumber = number; int numberOfDigits = (int)Math.Log10(number) + 1; int sum = 0; while (number > 0) { int digit = number % 10; sum += Power(digit, numberOfDigits); number /= 10; } return sum == originalNumber; } static void Main(string[] args) { Console.Write("Enter a number: "); int number = int.Parse(Console.ReadLine()); if (IsArmstrongNumber(number)) { Console.WriteLine($"{number} is an Armstrong number."); } else { Console.WriteLine($"{number} is not an Armstrong number."); } } }
How it Works
- Choose a Number: Start with any positive integer number. For example, let’s take the number 153.
- Determine the Number of Digits: Count how many digits are there in the chosen number. In our example, 153 has three digits.
- Split the Digits: Separate the number into its individual digits. For 153, you have 1, 5, and 3.
- Calculate the Power of Each Digit: Raise each digit to the power of the total number of digits in the original number. In our case, there are three digits, so we raise each digit to the power of 3.
- 1^3 = 1
- 5^3 = 125
- 3^3 = 27
- Sum the Cubes: Add up the results of these calculations.
- 1 + 125 + 27 = 153
- Compare with the Original Number: Compare the sum you obtained in the previous step with the original number you started with.
- Is 153 (sum) equal to 153 (original number)?
- Result: If the sum of the cubes of the digits is equal to the original number, then it’s an Armstrong number. In our example, 153 is indeed equal to the sum of its digits raised to the power of the number of digits, so it’s an Armstrong number.
Input/ Output
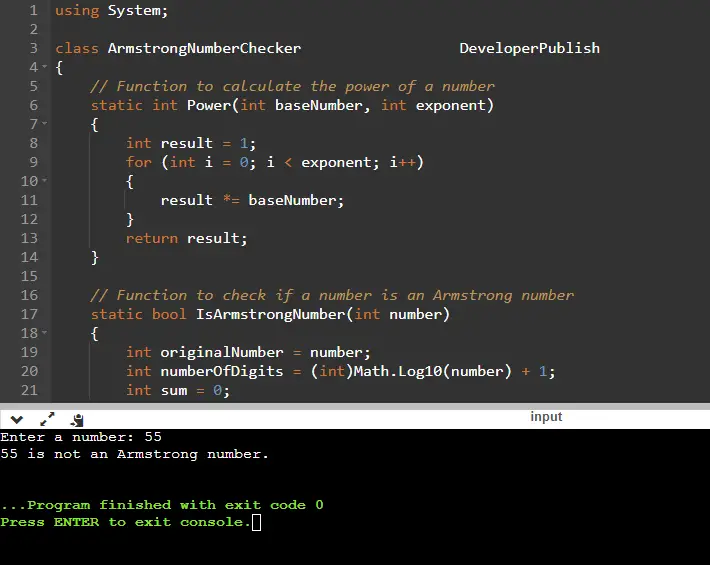