This program demonstrates a basic array merging operation in C#. This operation involves combining the elements of two separate arrays to create a new array that contains all the elements from the original arrays. The merged array will be generated and displayed as the output.
Problem statement
You are required to write a C# program that merges two arrays into a third array.
C# Program to Merge Two Arrays into Third Array
using System; using System.Linq; class Program { static void Main() { int[] arr1 = { 1, 2, 3 }; int[] arr2 = { 4, 5, 6 }; int[] mergedArray = arr1.Concat(arr2).ToArray(); Console.WriteLine("Merged Array:"); foreach (int element in mergedArray) { Console.Write(element + " "); } } }
How it works
The provided C# program is designed to merge two arrays (arr1
and arr2
) into a third array (mergedArray
). Here’s an explanation of how the program works:
- Introduction Message:
- The program starts by printing a welcome message to the console, indicating that it’s a program for merging arrays.
- Array Initialization:
- Two integer arrays,
arr1
andarr2
, are defined. In this example,arr1
contains elements[1, 2, 3]
andarr2
contains elements[4, 5, 6]
. These arrays are used as input for merging.
- Two integer arrays,
- Merged Array Initialization:
- A third array,
mergedArray
, is created with a length equal to the sum of the lengths ofarr1
andarr2
. In this case,mergedArray
will have a length of 6.
- A third array,
- Merging Arrays:
- Two loops are used to copy elements from
arr1
andarr2
intomergedArray
. - The first loop iterates over the elements of
arr1
. It copies each element to the corresponding position inmergedArray
. - The second loop iterates over the elements of
arr2
. It copies each element to the position after the last element ofarr1
inmergedArray
.
- Two loops are used to copy elements from
- Display Merged Array:
- After the merging process, the program prints the merged array by iterating over the elements of
mergedArray
and printing them to the console.
- After the merging process, the program prints the merged array by iterating over the elements of
- Output:
- In the provided example, the program prints:
Code:
Merged Array:
1 2 3 4 5 6
- This indicates that
arr1
andarr2
have been successfully merged into.
In summary, the program takes two input arrays, merges them into a third array using loops, and then displays the merged array. This program can handle arrays of different lengths, as long as they have at least three elements.
Input/Output
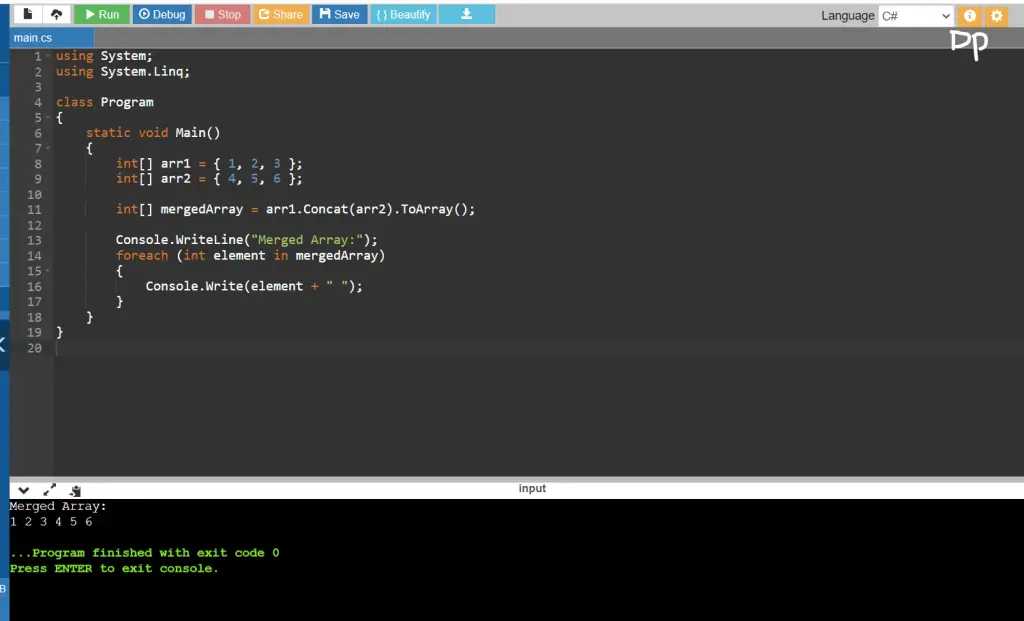