This program provides a simple yet effective way to determine if a given year is a leap year in C#. It’s a fundamental concept that can be a starting point for more complex date-related applications.
Problem statement
Write a C# program that determines whether a given year is a leap year or not.
C# Program to Check if a Given Year is a Leap Year
using System; class Program { static void Main() { Console.Write("Enter a year: "); int year = int.Parse(Console.ReadLine()); if (IsLeapYear(year)) { Console.WriteLine(year + " is a leap year."); } else { Console.WriteLine(year + " is not a leap year."); } } static bool IsLeapYear(int year) { // A year is a leap year if it is divisible by 4, // except for years that are divisible by 100 but not by 400. return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0); } }
How it works
The C# program provided checks if a given year is a leap year. Let’s break down how it works step by step:
User Input:
- The program starts by prompting the user to enter a year.
Code:
Console.Write(“Enter a year: “);
int year = int.Parse(Console.ReadLine());
The Console.Write
method displays the message “Enter a year: ” on the console, prompting the user to input a year. The Console.ReadLine
method reads the input as a string, and int.Parse
converts it to an integer, which is stored in the variable year
.
Leap Year Check:
- The program then calls the
IsLeapYear
method to determine if the entered year is a leap year.
Code:
if (IsLeapYear(year))
{
Console.WriteLine(year + ” is a leap year.”);
}
else
{
Console.WriteLine(year + ” is not a leap year.”);
}
If the IsLeapYear
method returns true
, it prints a message indicating that the year is a leap year. Otherwise, it prints a message indicating that it is not.
IsLeapYear
Method:
- This method contains the logic to determine if a year is a leap year.
Code:
static bool IsLeapYear(int year)
{
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
- The method takes an integer
year
as input and returns a boolean value (true
if it’s a leap year,false
if it’s not). - The logic checks two conditions:
year % 4 == 0 && year % 100 != 0
: This checks if the year is divisible by 4 but not divisible by 100. This is the general rule for leap years.year % 400 == 0
: This handles an exception to the general rule. If a year is divisible by 400, it is a leap year even if it’s also divisible by 100.
Output:
- Based on the result of the
IsLeapYear
method, the program prints an appropriate message indicating whether the year is a leap year or not.
Example Execution:
Let’s say the user inputs the year 2020
. The program will execute as follows:
- User Input:
2020
- Leap Year Check (
IsLeapYear(2020)
):(2020 % 4 == 0 && 2020 % 100 != 0)
evaluates totrue
.- So,
IsLeapYear(2020)
returnstrue
.
- Output:
2020 is a leap year.
The program will display this message on the console.
If the user inputs 1900
, the program will execute differently:
- User Input:
1900
- Leap Year Check (
IsLeapYear(1900)
):(1900 % 4 == 0 && 1900 % 100 != 0)
evaluates tofalse
.- So,
IsLeapYear(1900)
returnsfalse
.
- Output:
1900 is not a leap year.
This provides a clear indication of whether the entered year is a leap year or not.
Input/Output
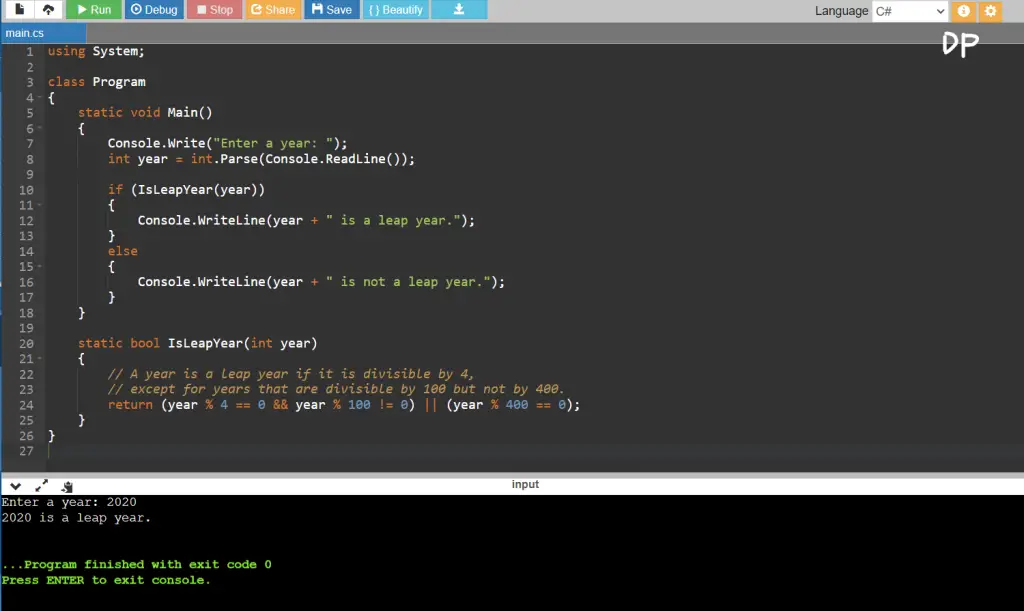