This C# program calculates and displays an upper triangular matrix based on user input.
Problem Statement:
The program takes the size of the matrix and the matrix elements as input and then generates and displays the upper triangular matrix.
C# Program to Display Upper Triangular Matrix
using System; class UpperTriangularMatrix { static void Main(string[] args) { Console.WriteLine("Upper Triangular Matrix Display"); Console.WriteLine("--------------------------------"); // Input the matrix size Console.Write("Enter the size of the matrix: "); int size = int.Parse(Console.ReadLine()); int[,] matrix = new int[size, size]; // Input the matrix elements Console.WriteLine("Enter the matrix elements:"); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { if (j >= i) { Console.Write($"Enter element at position [{i},{j}]: "); matrix[i, j] = int.Parse(Console.ReadLine()); } else { matrix[i, j] = 0; } } } // Display the upper triangular matrix Console.WriteLine("\nUpper Triangular Matrix:"); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { Console.Write(matrix[i, j] + " "); } Console.WriteLine(); } } }
How It Works:
- The program starts by asking the user to input the size of the matrix (number of rows and columns).
- It then creates a 2D array to store the matrix.
- Next, the program asks the user to input the elements of the matrix row by row, but it only accepts input for elements in the upper triangular part (where the column index is greater than or equal to the row index).
- For elements in the lower triangular part, the program sets them to zero.
- Finally, the program displays the upper triangular matrix.
Input/Output:
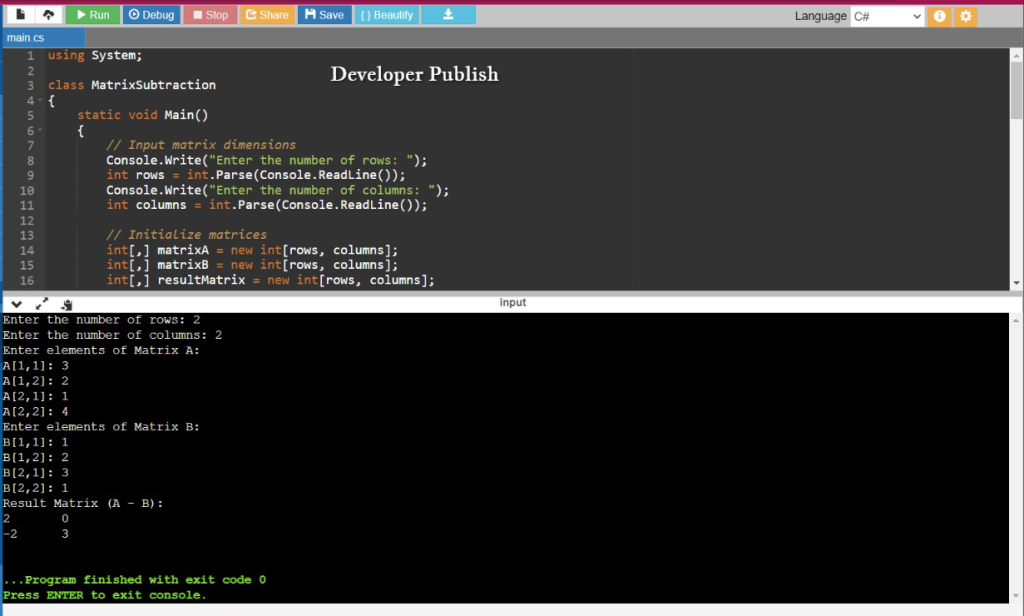