This C# program serves as a demonstration of bitwise operators, which are fundamental operators used for manipulating individual bits in integer values. Bitwise operators perform operations at the bit level, allowing you to work with binary representations of numbers. They are commonly used in low-level programming, embedded systems, and various computational tasks.
Problem Statement
Write a C# program to demonstrate bitwise operators. The program should take input from the user in the form of two integers. The program should display the result of each bitwise operation as output.
C# Program to Demonstrate Bitwise Operators
using System; class Program { static void Main(string[] args) { Console.WriteLine("Enter two numbers: "); int x = int.Parse(Console.ReadLine()); int y = int.Parse(Console.ReadLine()); int result = x & y; Console.WriteLine("{0} & {1} = {2}", x, y, result); result = x | y; Console.WriteLine("{0} | {1} = {2}", x, y, result); result = x ^ y; Console.WriteLine("{0} ^ {1} = {2}", x, y, result); result = ~x; Console.WriteLine("~{0} = {1}", x, result); result = x << 2; Console.WriteLine("{0} << 2 = {1}", x, result); result = x >> 2; Console.WriteLine("{0} >> 2 = {1}", x, result); } }
Input / Output
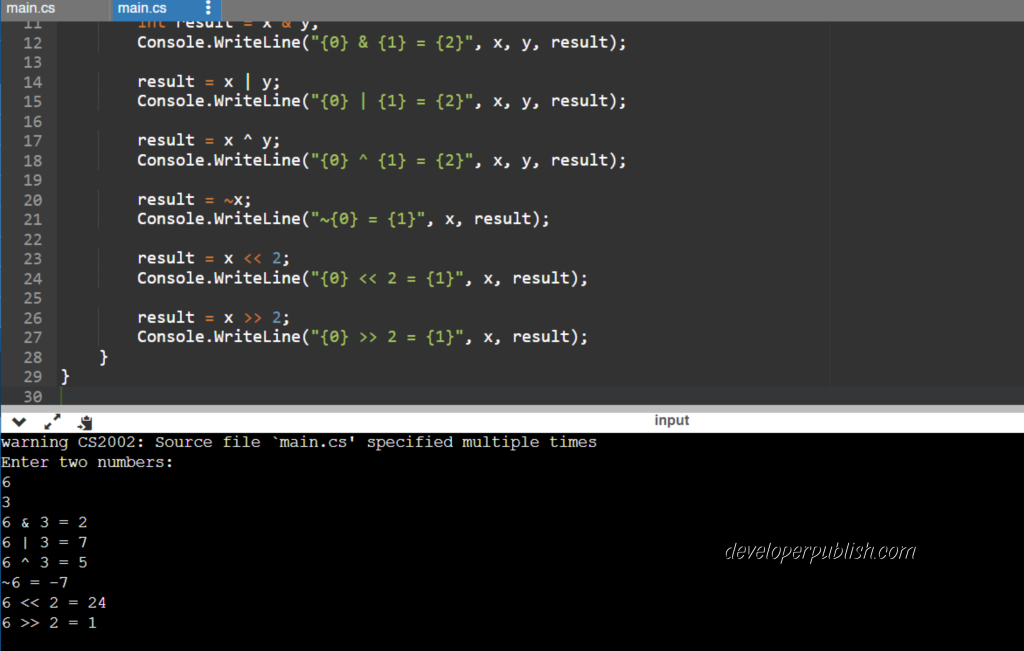