This C# program is designed to interchange or swap the columns of a matrix. A matrix is a two-dimensional array of numbers organized in rows and columns. Interchanging columns can be useful in various applications, such as matrix transformations, data manipulation, and sorting algorithms.
Problem Statement
Write a program that takes as input a matrix of integers and interchanges two specified columns of the matrix. The program should output the original matrix and the matrix with the columns interchanged.
C# Program to Interchange the Columns of a Matrix
using System; class MatrixInterchange { static void Main() { Console.Write("Enter the number of rows: "); int rows = int.Parse(Console.ReadLine()); Console.Write("Enter the number of columns: "); int columns = int.Parse(Console.ReadLine()); int[,] matrix = new int[rows, columns]; int[,] swappedMatrix = new int[rows, columns]; // Input matrix elements Console.WriteLine("Enter matrix elements:"); for (int i = 0; i < rows; i++) { for (int j = 0; j < columns; j++) { Console.Write($"Enter element at [{i},{j}]: "); matrix[i, j] = int.Parse(Console.ReadLine()); } } // Interchange columns Console.WriteLine("Matrix before column interchange:"); PrintMatrix(matrix); for (int i = 0; i < rows; i++) { for (int j = 0; j < columns; j++) { swappedMatrix[i, j] = matrix[i, columns - 1 - j]; } } Console.WriteLine("Matrix after column interchange:"); PrintMatrix(swappedMatrix); } static void PrintMatrix(int[,] matrix) { int rows = matrix.GetLength(0); int columns = matrix.GetLength(1); for (int i = 0; i < rows; i++) { for (int j = 0; j < columns; j++) { Console.Write(matrix[i, j] + " "); } Console.WriteLine(); } } }
How it Works
- Input Gathering:
- The program starts by prompting the user to enter the number of rows and columns for the matrix.
- Matrix Input:
- Next, the program asks the user to input the elements of the matrix row by row. It uses nested loops to read the values for each cell of the matrix.
- Column Indices Input:
- The program then prompts the user to enter the indices of the two columns they want to interchange. These indices are 1-based, meaning the first column is 1, the second is 2, and so on.
- Original Matrix Display:
- Before performing the interchange, the program displays the original matrix to the user.
- Column Interchange:
- Using another set of nested loops, the program creates a new matrix called
swappedMatrix
. - It iterates through each element of the original matrix and copies it to the corresponding position in the
swappedMatrix
. However, when copying columns, it swaps the order of the columns according to the specified indices. For example, if the user wants to interchange columns 2 and 4, it copies the element at (i, 2) in the original matrix to (i, 4) in theswappedMatrix
and vice versa.
- Using another set of nested loops, the program creates a new matrix called
- Display the Result:
- After performing the column interchange, the program displays the resulting matrix (i.e.,
swappedMatrix
) to the user, showing the effect of the column interchange.
- After performing the column interchange, the program displays the resulting matrix (i.e.,
- Program Completion:
- The program terminates after displaying the results.
Input/ Output
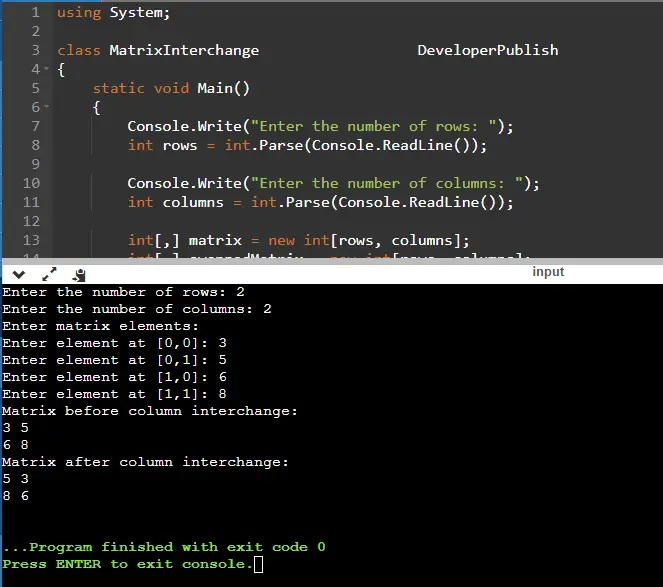