In this task, we will write a C# program to find the average of all the elements in an array. An array is a collection of variables of the same type that are referenced by a common name.
Problem Statement
Write a C# program to find the average of all the elements in an array. The program should take input from the user in the form of an array of integers. The program should display the average of the array elements as output.
C# Program to Find the Average of All the Array Elements
using System; class Program { static void Main(string[] args) { Console.WriteLine("Enter the number of elements in the array: "); int n = int.Parse(Console.ReadLine()); int[] arr = new int[n]; Console.WriteLine("Enter the elements of the array: "); for (int i = 0; i < n; i++) { arr[i] = int.Parse(Console.ReadLine()); } int sum = 0; for (int i = 0; i < n; i++) { sum += arr[i]; } double average = (double)sum / n; Console.WriteLine("The average of the array elements is {0}.", average); } }
Input / Output
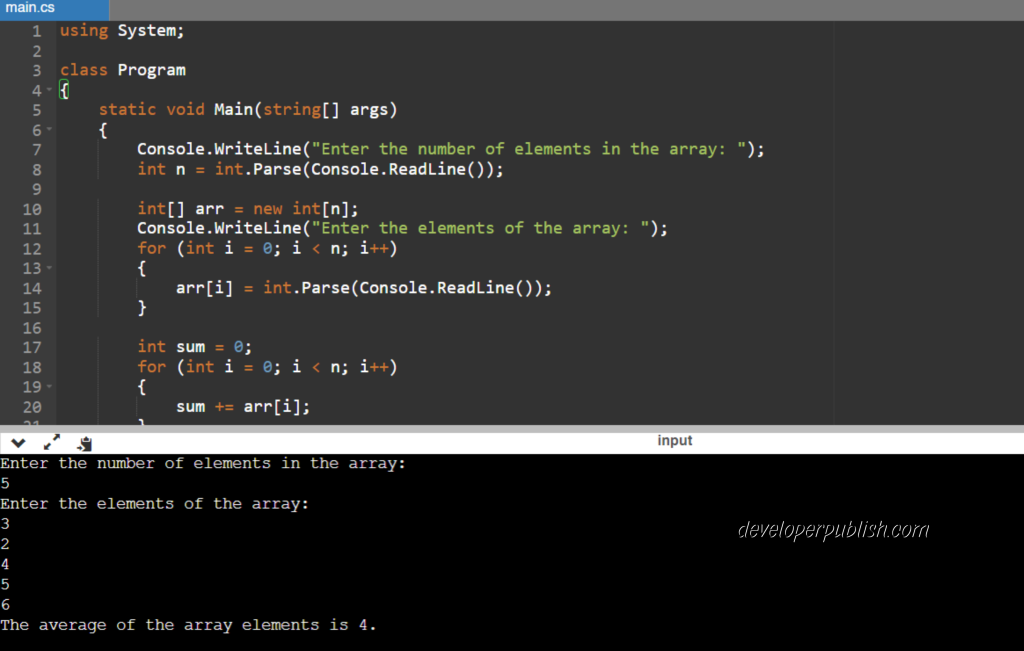